Bob Masters Advanced Shell Scripting on AlmaLinux
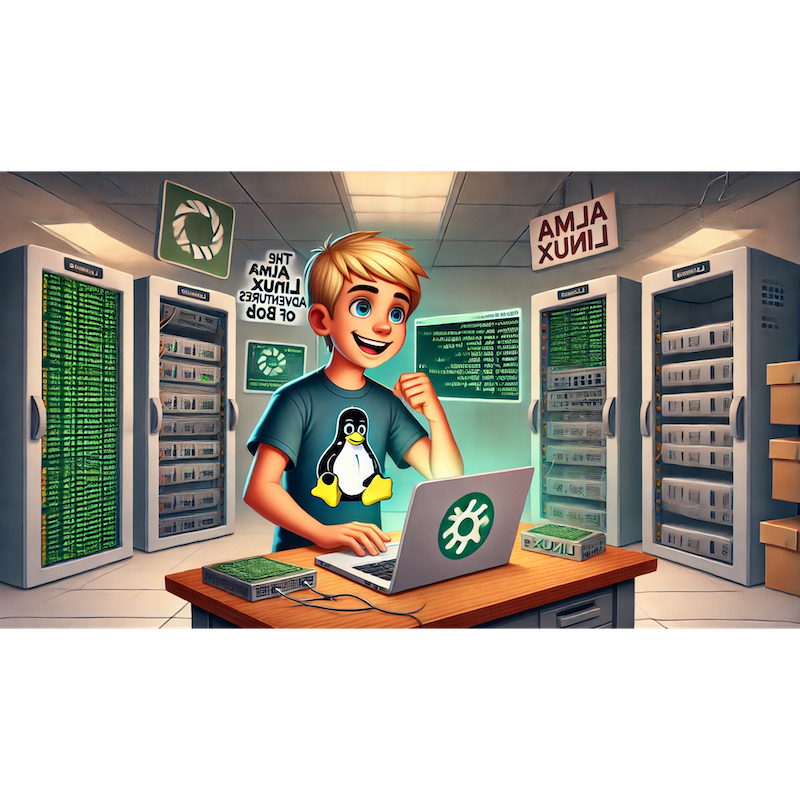
Categories:
3 minute read
Bob’s next challenge was to dive deeper into shell scripting, mastering techniques to automate complex workflows and optimize system administration. By writing advanced scripts, he aimed to save time, enhance precision, and solve problems efficiently.
“A good script is like a magic wand—time to craft some wizardry!” Bob said, excited to hone his scripting skills.
Chapter Outline: “Bob Masters Advanced Shell Scripting”
Introduction: Why Master Advanced Shell Scripting?
- The role of advanced scripts in Linux administration.
- Key benefits of scripting for automation and troubleshooting.
Exploring Advanced Shell Constructs
- Using functions and arrays for modular scripting.
- Leveraging conditionals and loops for dynamic workflows.
Working with Files and Processes
- Parsing and manipulating files with
awk
andsed
. - Managing processes and monitoring system states.
- Parsing and manipulating files with
Automating System Tasks
- Writing cron jobs and scheduled scripts.
- Automating backups and system updates.
Error Handling and Debugging
- Implementing error-handling mechanisms.
- Debugging scripts with
set
and logging.
Integrating Shell Scripts with Other Tools
- Combining shell scripts with Python or Ansible.
- Leveraging APIs and web services within scripts.
Conclusion: Bob Reflects on Scripting Mastery
Part 1: Why Master Advanced Shell Scripting?
Bob learned that advanced shell scripting is essential for:
- Automation: Handling repetitive tasks with precision.
- Optimization: Improving efficiency in system workflows.
- Troubleshooting: Quickly resolving complex issues.
Real-World Applications
- Managing log files and parsing data.
- Automating software installations.
- Monitoring and optimizing system performance.
“Scripting saves time and transforms tedious tasks into automated workflows!” Bob said.
Part 2: Exploring Advanced Shell Constructs
Step 1: Using Functions
Define reusable functions:
function greet_user() { echo "Hello, $1! Welcome to AlmaLinux." } greet_user "Bob"
Step 2: Working with Arrays
Use arrays to store and retrieve data:
servers=("web1" "web2" "db1") for server in "${servers[@]}"; do echo "Checking server: $server" done
Step 3: Dynamic Workflows with Conditionals and Loops
Write dynamic scripts:
if [ -f "/etc/passwd" ]; then echo "File exists." else echo "File not found!" fi
“Functions and arrays make scripts modular and dynamic!” Bob noted.
Part 3: Working with Files and Processes
Step 1: Parsing Files with awk
and sed
Extract specific columns from a file:
awk -F: '{print $1, $3}' /etc/passwd
Replace text in a file:
sed -i 's/oldtext/newtext/g' file.txt
Step 2: Managing Processes
Monitor resource-hungry processes:
ps aux --sort=-%mem | head
Kill a process by name:
pkill -f "process_name"
“File parsing and process management are powerful troubleshooting tools!” Bob said.
Part 4: Automating System Tasks
Step 1: Writing Cron Jobs
- Automate backups with a cron job:
Create a script:
#!/bin/bash tar -czf /backup/home_backup.tar.gz /home/bob
Schedule the script:
crontab -e
Add:
0 2 * * * /home/bob/backup.sh
Step 2: Automating Updates
Write a script to automate updates:
#!/bin/bash sudo dnf update -y
Schedule the update script with cron:
0 3 * * 7 /home/bob/update.sh
“Scheduled scripts handle tasks without manual intervention!” Bob said.
Part 5: Error Handling and Debugging
Step 1: Implementing Error Handling
Use traps to catch errors:
trap 'echo "An error occurred. Exiting..."; exit 1' ERR
Step 2: Debugging with set
Enable debugging:
set -x
Log script output:
./script.sh > script.log 2>&1
“Error handling and debugging make scripts reliable and robust!” Bob noted.
Part 6: Integrating Shell Scripts with Other Tools
Step 1: Combining with Python
Call a Python script from a shell script:
python3 analyze_data.py input.csv
Step 2: Leveraging APIs
Use
curl
to interact with web services:curl -X GET "https://api.example.com/data" -H "Authorization: Bearer TOKEN"
“Shell scripts can integrate seamlessly with other tools for greater functionality!” Bob said.
Conclusion: Bob Reflects on Scripting Mastery
Bob mastered advanced shell scripting techniques, automating tasks, managing files and processes, and integrating scripts with other tools. By debugging and optimizing his scripts, he felt confident handling complex workflows in AlmaLinux.
Next, Bob plans to explore Linux Security Best Practices, ensuring robust protection for his systems.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.