Boolean Functions and Expressions: A Comprehensive Guide
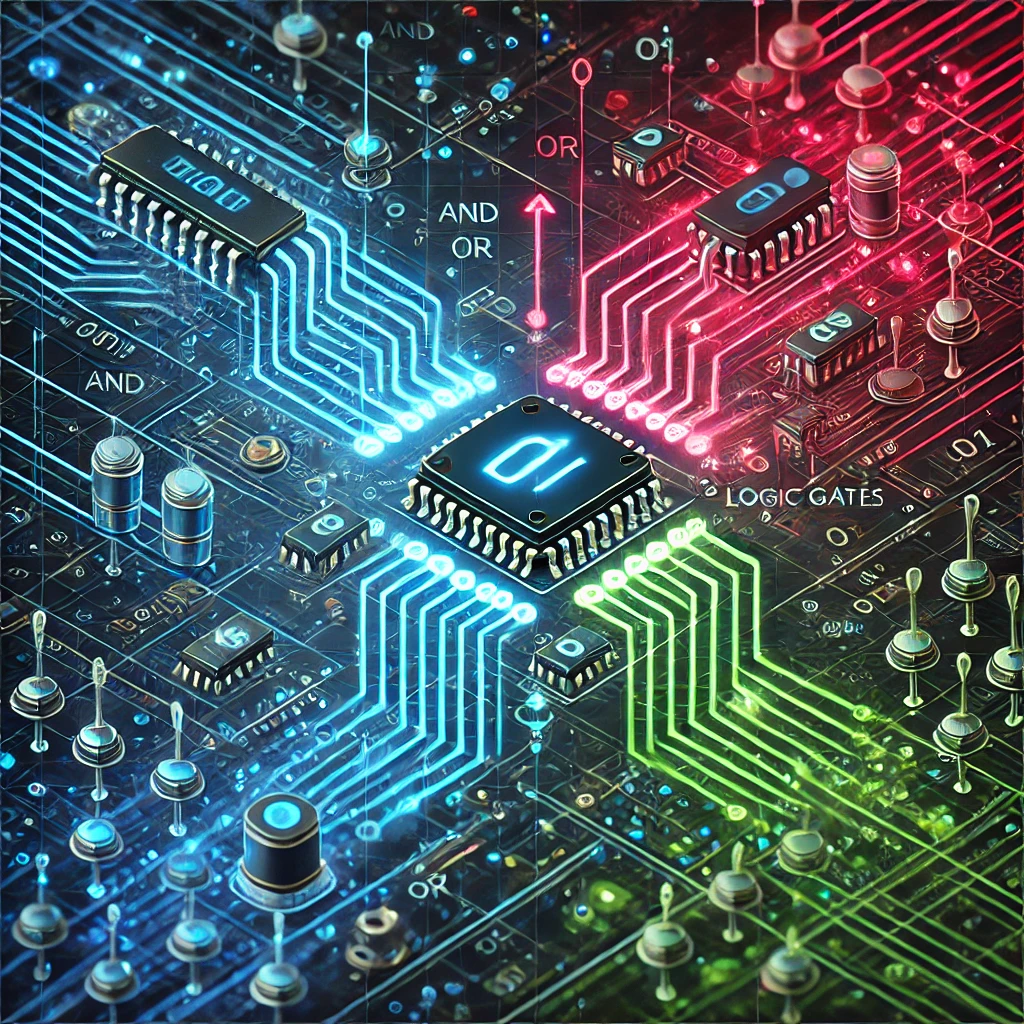
Categories:
4 minute read
Introduction
In the realm of computer science, Boolean logic, named after the mathematician George Boole, provides a fundamental framework for representing and manipulating logical statements. Boolean functions and expressions form the cornerstone of this logic, enabling us to express and evaluate conditions, make decisions, and control the flow of information within computer programs.
Understanding Boolean Values
At the heart of Boolean logic are Boolean values, which can only be either true or false. These values represent the outcomes of logical conditions or expressions. For instance, the statement “2 is greater than 1” is a Boolean expression that evaluates to true, while the statement “5 is less than 3” evaluates to false.
Boolean Operations
To combine and manipulate Boolean values, we employ Boolean operations. The three primary Boolean operations are:
AND (
&&
orAND
): The AND operation returns true only if both operands are true. Otherwise, it returns false.OR (
||
orOR
): The OR operation returns true if at least one operand is true. It returns false only if both operands are false.NOT (
!
orNOT
): The NOT operation negates the value of its operand. It returns true if the operand is false, and vice versa. Boolean Expressions
Boolean expressions are formed by combining Boolean values and variables using Boolean operations. They are used to represent logical conditions and evaluate to either true or false. Here are some examples of Boolean expressions:
(x > 5) AND (y < 10)
NOT (z = 0)
(a OR b) AND (c OR d)
Truth Tables
A truth table is a tabular representation of the possible combinations of input values and the corresponding output values for a Boolean function. It is a valuable tool for understanding and analyzing the behavior of Boolean expressions.
Input A | Input B | AND | OR | NOT A |
---|---|---|---|---|
0 | 0 | 0 | 0 | 1 |
0 | 1 | 0 | 1 | 1 |
1 | 0 | 0 | 1 | 0 |
1 | 1 | 1 | 1 | 0 |
Boolean Functions
A Boolean function is a mathematical function that maps a set of Boolean inputs to a single Boolean output. It can be represented using a truth table or a Boolean expression.
Examples of Boolean Functions
AND function:
f(A, B) = A AND B
OR function:
f(A, B) = A OR B
NOT function:
f(A) = NOT A
XOR (exclusive OR) function:
f(A, B) = (A OR B) AND NOT (A AND B)
Applications of Boolean Functions and Expressions
Boolean logic has widespread applications in various fields, including:
Computer hardware: Digital circuits and logic gates are designed based on Boolean functions.
Programming: Boolean expressions are used to control the flow of execution in programming languages.
Database systems: Boolean operators are used for query optimization and retrieval.
Artificial intelligence: Boolean logic is employed in knowledge representation and reasoning. Boolean Algebra
Boolean algebra is a mathematical system that provides a framework for manipulating and simplifying Boolean expressions. It is based on a set of axioms and rules that govern the behavior of Boolean operations.
Boolean Algebra Laws
Commutative laws:*
A AND B = B AND A
A OR B = B OR A
Associative laws:*
(A AND B) AND C = A AND (B AND C)
(A OR B) OR C = A OR (B OR C)
Distributive laws:*
A AND (B OR C) = (A AND B) OR (A AND C)
A OR (B AND C) = (A OR B) AND (A OR C)
Identity laws:*
A AND 1 = A
A OR 0 = A
Complement laws:*
A AND NOT A = 0
A OR NOT A = 1
De Morgan’s laws:*
NOT (A AND B) = NOT A OR NOT B
NOT (A OR B) = NOT A AND NOT B
Simplifying Boolean Expressions
By applying Boolean algebra laws, we can simplify complex Boolean expressions into equivalent but simpler forms. This simplification can improve the efficiency of digital circuits and reduce the computational overhead in software applications.
Karnaugh Maps
Karnaugh maps are a graphical tool used to simplify Boolean expressions. They provide a visual representation of the truth table, making it easier to identify and group adjacent cells that have the same output value.
Conclusion
Boolean functions and expressions are fundamental building blocks of computer science. They provide a powerful framework for representing and manipulating logical statements, enabling us to make decisions, control the flow of information, and design complex systems. Understanding Boolean logic is essential for anyone working in fields such as computer engineering, computer science, and digital electronics.