Closures in Kotlin Programming Language
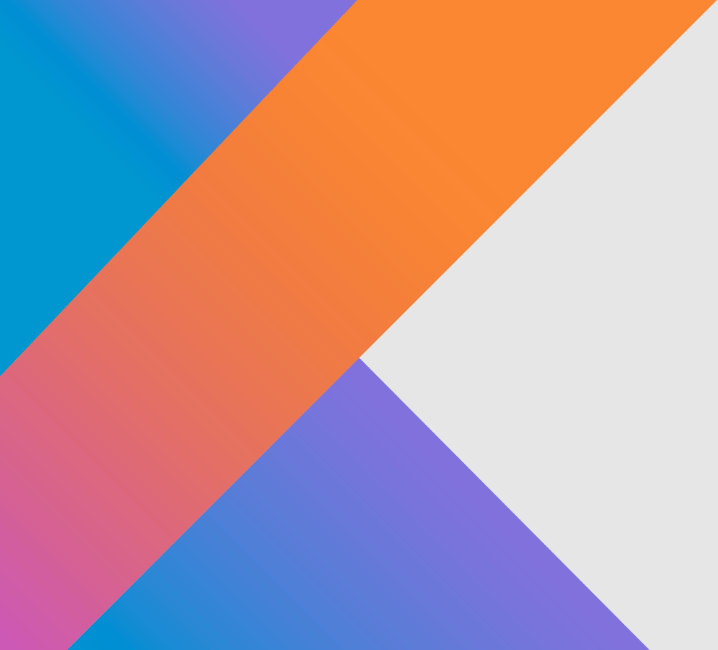
Categories:
5 minute read
Introduction
Kotlin is a modern, expressive programming language that runs on the Java Virtual Machine (JVM) and is widely used for Android development, backend services, and even multi-platform applications. One of its powerful features is the concept of closures, which play a crucial role in functional programming and higher-order functions. Closures allow developers to write cleaner, more concise, and more efficient code, especially when working with lambda expressions and anonymous functions.
This blog post will provide a comprehensive overview of closures in Kotlin, explaining their purpose, how they work, and their practical applications. By the end of this article, you will have a deep understanding of closures and how to use them effectively in your Kotlin programs.
What is a Closure?
A closure is a function that captures variables from its surrounding scope, allowing those variables to persist even after their original scope has ended. This concept enables functions to maintain state and be used in a more flexible manner, particularly in functional programming.
Key Characteristics of Closures
- Captures variables from an outer function – A closure can access and modify variables declared outside of its immediate scope.
- Retains variable values – Even after the outer function has finished execution, the captured variables persist in memory.
- Useful for functional programming – Closures work seamlessly with higher-order functions, allowing for more expressive and compact code.
Closures in Kotlin: The Basics
Kotlin provides multiple ways to create closures using lambda expressions and anonymous functions. These function types allow capturing variables from their surrounding scope.
Example of a Simple Closure
fun main() {
var counter = 0 // Variable in the outer scope
val increment = { counter++ } // Lambda capturing counter
increment()
increment()
println("Counter: $counter") // Output: Counter: 2
}
In this example:
counter
is a variable defined in the outer function.increment
is a lambda expression that incrementscounter
.- Even though
increment
runs independently, it retains access tocounter
and modifies it.
Anonymous Functions as Closures
Closures can also be created using anonymous functions, which are another way of defining functions without explicitly naming them.
fun main() {
var message = "Hello"
val changeMessage = fun() {
message = "Hello, Kotlin!"
}
changeMessage()
println(message) // Output: Hello, Kotlin!
}
Here, changeMessage
is an anonymous function that modifies message
, capturing it from the surrounding scope.
Closures and Higher-Order Functions
Higher-order functions are functions that accept other functions as parameters or return them as results. Closures work seamlessly with higher-order functions, making Kotlin’s functional programming paradigm more powerful.
Example: Using Closures with Higher-Order Functions
fun createMultiplier(factor: Int): (Int) -> Int {
return { number -> number * factor } // Lambda capturing factor
}
fun main() {
val double = createMultiplier(2)
val triple = createMultiplier(3)
println(double(5)) // Output: 10
println(triple(5)) // Output: 15
}
In this example:
createMultiplier
is a higher-order function that returns a lambda function.- The returned lambda function captures
factor
, enabling it to retain its value even aftercreateMultiplier
has executed. - The closure allows us to generate specialized functions like
double
andtriple
.
Mutable and Immutable Captured Variables
When a closure captures a variable, its behavior depends on whether the variable is mutable or immutable.
Capturing a Mutable Variable
fun main() {
var count = 0
val increment = { count++ }
increment()
increment()
println("Count: $count") // Output: Count: 2
}
Since count
is mutable, the closure modifies it directly, and the changes persist across function calls.
Capturing an Immutable Variable
fun main() {
val greeting = "Hello"
val closure = { println(greeting) }
closure() // Output: Hello
}
Here, the closure captures the immutable greeting
variable and can use it within its scope, but it cannot modify it.
Common Use Cases of Closures in Kotlin
Closures are used extensively in real-world Kotlin programming. Here are some common scenarios where closures shine:
1. Callbacks and Event Listeners
Closures are commonly used for implementing callbacks in asynchronous programming, such as handling user inputs or API responses.
fun fetchData(callback: (String) -> Unit) {
callback("Data received")
}
fun main() {
fetchData { data -> println(data) } // Output: Data received
}
2. Custom Sorting Functions
Closures can be used in sorting functions to define custom sorting logic.
fun main() {
val numbers = listOf(5, 3, 8, 1, 2)
val sortedNumbers = numbers.sortedBy { it }
println(sortedNumbers) // Output: [1, 2, 3, 5, 8]
}
3. Memoization and Caching
Closures enable caching of results to optimize performance.
fun memoizedAdder(): (Int) -> Int {
val cache = mutableMapOf<Int, Int>()
return { n ->
cache.getOrPut(n) { n + 10 }
}
}
fun main() {
val add10 = memoizedAdder()
println(add10(5)) // Output: 15
println(add10(5)) // Cached result: 15
}
Conclusion
Closures are a fundamental concept in Kotlin that enable functions to retain access to variables outside their immediate scope. By leveraging closures, developers can write more expressive and efficient code, particularly in functional programming and higher-order functions.
In this blog post, we explored:
- What closures are and how they work in Kotlin.
- How to use lambda expressions and anonymous functions to create closures.
- The interaction of closures with higher-order functions.
- Real-world applications of closures in sorting, callbacks, and memoization.
Understanding closures is essential for mastering Kotlin’s functional programming paradigm. Whether you’re developing Android applications, backend services, or working with Kotlin multi-platform projects, closures will be a powerful tool in your coding arsenal.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.