Common data types in C++
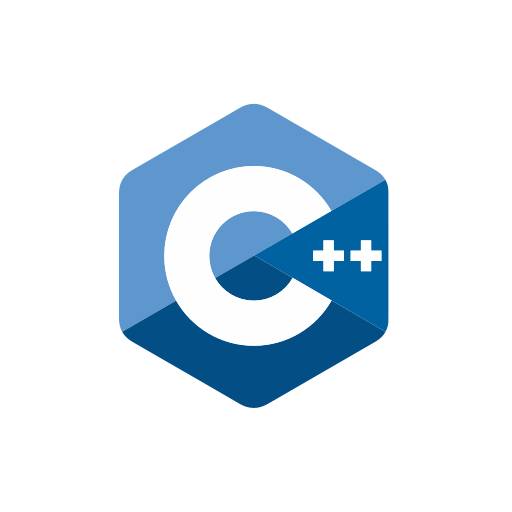
Categories:
less than a minute
C++ supports several different data types. Here are some of the most common ones:
Integer types (
int
): These are used to store whole numbers. The size of anint
is usually 4 bytes (32 bits), and it can store numbers from -2,147,483,648 to 2,147,483,647.Floating-point types (
float
,double
): These are used to store real numbers (numbers with fractional parts). Afloat
typically occupies 4 bytes of memory, while adouble
occupies 8 bytes.Character types (
char
): These are used to store individual characters. Achar
occupies 1 byte of memory and can store any character in the ASCII table.Boolean type (
bool
): This type is used to store eithertrue
orfalse
.String type (
std::string
): This is used to store sequences of characters, or strings. It’s not a built-in type, but is included in the C++ Standard Library.Array types: These are used to store multiple values of the same type in a single variable.
Pointer types: These are used to store memory addresses.
User-defined types (classes, structs, unions, enums): These allow users to define their own data types. Each of these types has its own characteristics and uses, and understanding them is fundamental to programming in C++.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.