Companion Objects in Kotlin
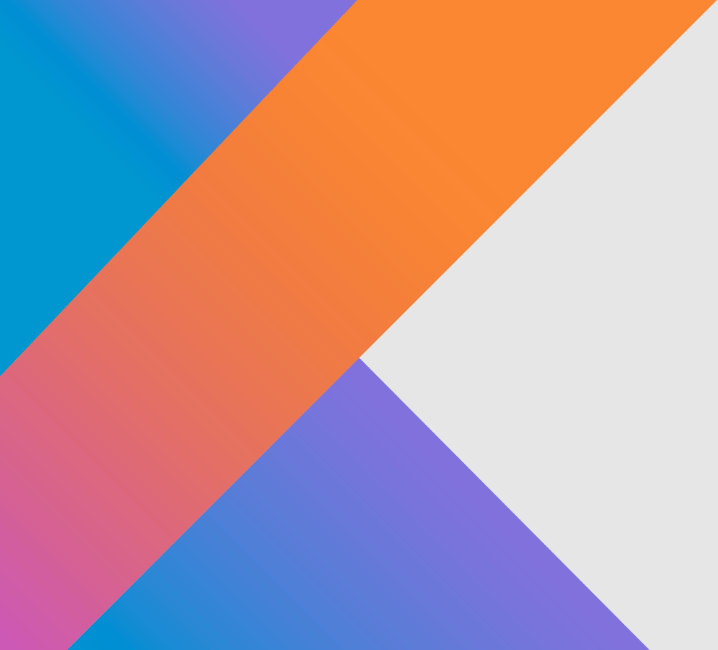
Categories:
5 minute read
Companion objects are a fundamental feature of Kotlin that provide a sophisticated way to handle static members and factory patterns. In this comprehensive guide, we’ll explore how companion objects work, their capabilities, and best practices for using them effectively in your Kotlin applications.
Understanding Companion Objects
A companion object is a special object declaration inside a class that is marked with the companion
keyword. It allows you to define members that are tied to the class itself rather than to instances of the class, similar to static members in Java but with more flexibility and features.
Basic Syntax and Usage
Let’s start with the basic syntax of companion objects:
class PaymentGateway {
companion object {
const val API_VERSION = "v1.0"
fun create(config: Map<String, String>): PaymentGateway {
return PaymentGateway().apply {
// Initialize with config
}
}
}
fun processPayment(amount: Double) {
// Instance method implementation
}
}
You can access companion object members directly through the class name:
val version = PaymentGateway.API_VERSION
val gateway = PaymentGateway.create(mapOf("key" to "value"))
Key Features of Companion Objects
Named Companion Objects
While companion objects can be anonymous, you can also give them names:
class DatabaseConnection {
companion object Factory {
fun create(url: String): DatabaseConnection {
// Connection creation logic
return DatabaseConnection()
}
}
}
The name can be useful when implementing interfaces or for clarity in larger codebases:
val connection = DatabaseConnection.Factory.create("jdbc:postgresql://localhost:5432/db")
Interface Implementation
Companion objects can implement interfaces, making them powerful tools for factory patterns:
interface ConnectionFactory {
fun create(url: String): DatabaseConnection
}
class DatabaseConnection {
companion object : ConnectionFactory {
override fun create(url: String): DatabaseConnection {
return DatabaseConnection()
}
}
}
Extension Functions
One of the unique features of companion objects is that you can define extension functions for them:
class Logger {
companion object {
fun log(message: String) {
println(message)
}
}
}
fun Logger.Companion.debug(message: String) {
log("[DEBUG] $message")
}
// Usage
Logger.debug("This is a debug message")
Common Use Cases and Patterns
Factory Pattern Implementation
Companion objects are perfect for implementing factory patterns:
sealed class Response {
data class Success(val data: String) : Response()
data class Error(val message: String) : Response()
companion object {
fun success(data: String) = Success(data)
fun error(message: String) = Error(message)
fun from(responseCode: Int, data: String): Response {
return when (responseCode) {
200 -> success(data)
else -> error("Error: $responseCode")
}
}
}
}
Constants and Configuration
Companion objects provide a clean way to define class-level constants and configuration:
class NetworkClient {
companion object {
const val DEFAULT_TIMEOUT = 30000L
const val DEFAULT_RETRY_COUNT = 3
private val VALID_PROTOCOLS = setOf("http", "https")
fun isValidProtocol(protocol: String): Boolean {
return protocol.lowercase() in VALID_PROTOCOLS
}
}
}
Singleton Pattern with Additional Functionality
While Kotlin’s object declaration is the primary way to implement singletons, companion objects can enhance singleton-like classes with additional functionality:
class ApplicationConfig private constructor() {
var debug: Boolean = false
var environment: String = "development"
companion object {
private var instance: ApplicationConfig? = null
fun getInstance(): ApplicationConfig {
return instance ?: synchronized(this) {
instance ?: ApplicationConfig().also { instance = it }
}
}
fun reset() {
instance = null
}
}
}
Best Practices and Guidelines
Encapsulation and Privacy
Use private constructors with companion object factories to enforce proper object creation:
class SecureConnection private constructor(private val config: ConnectionConfig) {
companion object {
fun create(config: ConnectionConfig): SecureConnection {
require(config.isValid()) { "Invalid configuration" }
return SecureConnection(config)
}
}
}
Separation of Concerns
Keep companion object methods focused and related to the class they’re associated with:
class UserRepository {
companion object {
// Good: Factory methods related to UserRepository
fun createWithDatabase(database: Database): UserRepository {
return UserRepository()
}
// Bad: Unrelated utility function
fun formatDate(date: Date): String { // This should be in a DateUtils object
return SimpleDateFormat("yyyy-MM-dd").format(date)
}
}
}
Documentation
Always document companion object members, especially when they’re part of your public API:
class ApiClient {
companion object {
/**
* Creates an ApiClient instance with the specified configuration.
* @param config The configuration object containing API credentials
* @throws IllegalArgumentException if the configuration is invalid
*/
fun create(config: ApiConfig): ApiClient {
require(config.apiKey.isNotBlank()) { "API key cannot be blank" }
return ApiClient()
}
}
}
Performance Considerations
Companion objects are initialized lazily when they’re first accessed. This means they don’t impact your application’s startup time unless they’re actually used:
class HeavyProcessor {
companion object {
// This initialization only happens when the companion object is first accessed
private val heavyResource = loadHeavyResource()
private fun loadHeavyResource(): Resource {
// Expensive initialization
return Resource()
}
}
}
Testing Considerations
When testing classes with companion objects, consider these approaches:
class ProductService {
companion object {
var idGenerator: () -> String = { UUID.randomUUID().toString() }
fun createProduct(name: String): Product {
return Product(idGenerator(), name)
}
}
}
// In tests
fun testProductCreation() {
ProductService.idGenerator = { "fixed-id" }
val product = ProductService.createProduct("Test Product")
assertEquals("fixed-id", product.id)
}
Conclusion
Companion objects are a powerful feature in Kotlin that provide a clean and flexible way to implement class-level functionality. They offer advantages over traditional static members while maintaining readability and type safety. By following best practices and understanding their capabilities, you can use companion objects effectively to create more maintainable and elegant code.
Remember that while companion objects are powerful, they should be used judiciously. Consider whether functionality truly belongs at the class level, and be mindful of testing implications when using companion objects in your code. With proper usage, companion objects can significantly improve your Kotlin codebase’s organization and design.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.