Create variables and assign values to them in C++
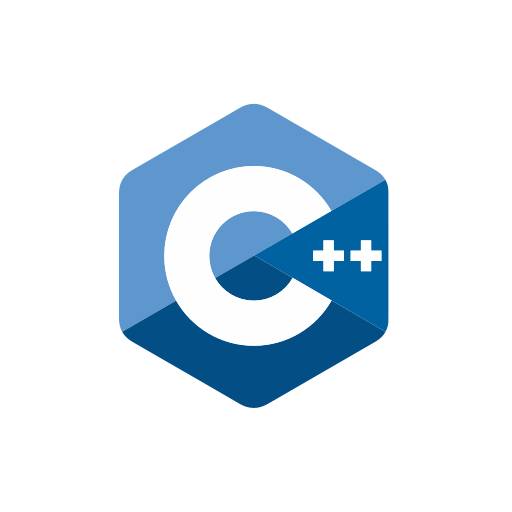
Categories:
2 minute read
In C++, you can create variables and assign values to them in the following way:
Declare a variable by specifying its type followed by the variable name. For example,
int myVariable;
declares a variable namedmyVariable
of typeint
.Assign a value to the variable using the assignment operator
=
. For example,myVariable = 5;
assigns the value5
tomyVariable
. Here is an example of creating different types of variables and assigning values to them:
// Include the necessary libraries
#include <iostream> // for input/output operations
#include <string> // for using string data type
// Main function where the program starts execution
int main() {
// Declare an integer variable
int myInt;
// Assign a value to the integer variable
myInt = 10;
// Declare a double variable and assign a value to it
double myDouble = 20.5;
// Declare a character variable and assign a value to it
char myChar = 'A';
// Declare a string variable and assign a value to it
std::string myString = "Hello, World!";
// Declare a boolean variable and assign a value to it
bool myBool = true;
// End of main function, return 0 to indicate successful execution
return 0;
}
Explanation
The provided code is a simple C++ program that demonstrates the declaration and initialization of variables of different types.
The program begins by including necessary libraries. The iostream
library is included for input/output operations, and the string
library is used to handle string data types.
#include <iostream> // for input/output operations
#include <string> // for using string data type```
The `main` function is where the program starts execution. Inside this function, several variables of different types are declared and initialized.
```cpp
int main() {
...
return 0;
}
An integer variable myInt
is declared and then assigned a value of 10
.
int myInt;
myInt = 10;
A double variable myDouble
is declared and assigned a value of 20.5
in the same line.
double myDouble = 20.5;
Similarly, a character variable myChar
is declared and assigned the character ‘A’.
char myChar = 'A';
A string variable myString
is declared and assigned the string “Hello, World!”.
std::string myString = "Hello, World!";
Lastly, a boolean variable myBool
is declared and assigned the value true
.
bool myBool = true;
The function ends with a return 0;
statement, indicating successful execution of the program. As it stands, the program does not produce any output. It simply demonstrates how to declare and initialize variables of different types in C++.