Dispatchers in Kotlin Programming Language
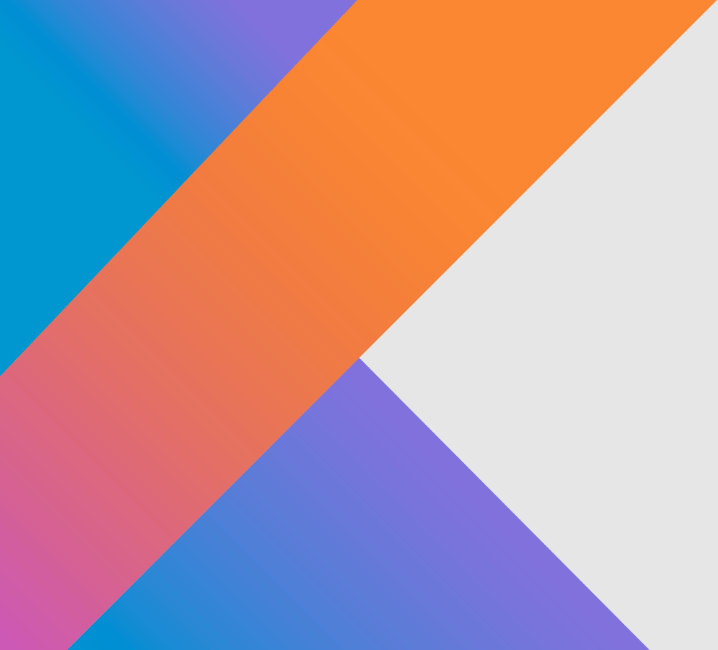
Categories:
4 minute read
Introduction
Kotlin is a modern, expressive, and concise programming language that has gained immense popularity, especially in Android development. One of Kotlin’s most powerful features is coroutines, which allow developers to write asynchronous code in a sequential and readable manner.
When working with coroutines in Kotlin, Dispatchers play a crucial role in determining which thread a coroutine will execute on. Understanding Dispatchers is essential for optimizing application performance, improving responsiveness, and ensuring efficient multitasking.
In this article, we will explore the concept of Dispatchers in Kotlin, how they work, and best practices for using them effectively.
What Are Dispatchers in Kotlin?
In Kotlin Coroutines, a Dispatcher is responsible for assigning coroutines to different threads. It determines where and how the coroutines will run—whether on the main thread, background thread, or a new thread.
Kotlin provides different types of Dispatchers, each optimized for specific use cases. These include:
Dispatchers.Main
– Runs coroutines on the main UI thread.Dispatchers.IO
– Optimized for I/O operations like file reading and network calls.Dispatchers.Default
– Best for CPU-intensive operations.Dispatchers.Unconfined
– Starts the coroutine in the current thread but can switch later.
Let’s dive into each of these in detail.
Types of Dispatchers in Kotlin
1. Dispatchers.Main
The Dispatchers.Main
is specifically designed for running coroutines on the main UI thread. It is primarily used in Android applications to update UI components safely.
Example Usage:
import kotlinx.coroutines.*
fun main() {
GlobalScope.launch(Dispatchers.Main) {
println("Running on the main thread: ${Thread.currentThread().name}")
}
}
However, Dispatchers.Main
is only available in environments that support a main UI thread, like Android.
When to Use?
- Updating UI components
- Handling user interactions
- Running lightweight UI tasks
Best Practices:
- Avoid performing heavy operations on
Dispatchers.Main
, as it may block the UI and make the app unresponsive.
2. Dispatchers.IO
The Dispatchers.IO
is designed for background I/O operations such as:
- Reading/writing files
- Making network requests
- Accessing databases
Since these tasks can be time-consuming, Dispatchers.IO
runs them on a separate thread pool, preventing them from blocking the UI thread.
Example Usage:
GlobalScope.launch(Dispatchers.IO) {
val data = fetchDataFromNetwork()
println("Data fetched on: ${Thread.currentThread().name}")
}
Here, fetchDataFromNetwork()
executes on a background thread without affecting the UI.
When to Use?
- Performing file operations
- Fetching API data from a server
- Reading/writing to a database
Best Practices:
- Use
Dispatchers.IO
only for I/O-bound tasks to avoid unnecessary thread switching. - Combine with
withContext(Dispatchers.Main)
to update UI after fetching data.
3. Dispatchers.Default
The Dispatchers.Default
is used for CPU-intensive operations that require significant processing power. It is optimized for tasks like:
- Sorting large datasets
- Complex mathematical calculations
- Image processing
Since these operations are CPU-bound, Kotlin assigns them to a separate thread pool for better efficiency.
Example Usage:
GlobalScope.launch(Dispatchers.Default) {
val result = heavyComputation()
println("Computation completed on: ${Thread.currentThread().name}")
}
Here, heavyComputation()
runs in a background thread to avoid blocking the main thread.
When to Use?
- Complex data processing
- Running algorithms that require intensive computations
Best Practices:
- Avoid using
Dispatchers.Default
for simple tasks, as it might consume unnecessary resources. - Keep CPU-intensive tasks short to prevent blocking threads.
4. Dispatchers.Unconfined
The Dispatchers.Unconfined
starts a coroutine in the current thread and continues execution in the same thread unless it encounters a suspending function, which may change its execution context.
Example Usage:
GlobalScope.launch(Dispatchers.Unconfined) {
println("Before suspension: ${Thread.currentThread().name}")
delay(1000) // This may change the thread
println("After suspension: ${Thread.currentThread().name}")
}
Since the execution may shift to another thread after delay(1000)
, Dispatchers.Unconfined
is generally unpredictable.
When to Use?
- Rare cases when thread switching is unnecessary
- Quick prototyping/testing
Best Practices:
- Avoid using
Dispatchers.Unconfined
in production as it may lead to unpredictable behavior. - Use it only when you don’t need a specific thread assignment.
Switching Between Dispatchers
Sometimes, you may need to switch between Dispatchers within a coroutine. The withContext()
function helps achieve this efficiently.
Example Usage:
GlobalScope.launch(Dispatchers.IO) {
val data = fetchData()
withContext(Dispatchers.Main) {
updateUI(data)
}
}
Here, fetchData()
runs on a background thread (Dispatchers.IO
), and updateUI(data)
runs on the UI thread (Dispatchers.Main
).
Custom Coroutine Dispatcher
If the predefined Dispatchers (Main
, IO
, Default
) don’t meet your needs, you can create a custom Coroutine Dispatcher using newSingleThreadContext()
.
Example Usage:
val customDispatcher = newSingleThreadContext("MyThread")
GlobalScope.launch(customDispatcher) {
println("Running on: ${Thread.currentThread().name}")
}
This approach is useful for tasks that require exclusive access to a dedicated thread.
Best Practices:
- Avoid excessive creation of custom Dispatchers, as it may consume system resources.
- Always close custom Dispatchers using
close()
to free up resources.
Comparing Different Dispatchers
Dispatcher | Best for | Example Use Cases |
---|---|---|
Dispatchers.Main | UI updates | Displaying data in a TextView |
Dispatchers.IO | I/O operations | Making API requests |
Dispatchers.Default | CPU-intensive tasks | Sorting large data sets |
Dispatchers.Unconfined | Quick testing | Debugging coroutines |
Conclusion
Understanding Dispatchers in Kotlin is essential for writing efficient, responsive, and scalable applications. Each Dispatcher serves a unique purpose:
- Use
Dispatchers.Main
for UI-related tasks. - Use
Dispatchers.IO
for network and file operations. - Use
Dispatchers.Default
for CPU-heavy computations. - Avoid
Dispatchers.Unconfined
in production due to unpredictable behavior.
By using the right Dispatcher for the right task, developers can maximize application performance and responsiveness.
With this knowledge, you can now leverage Kotlin coroutines effectively in your projects. Happy coding! 🚀
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.