Divide-and-Conquer Method in Computer Algorithms: A Comprehensive Guide
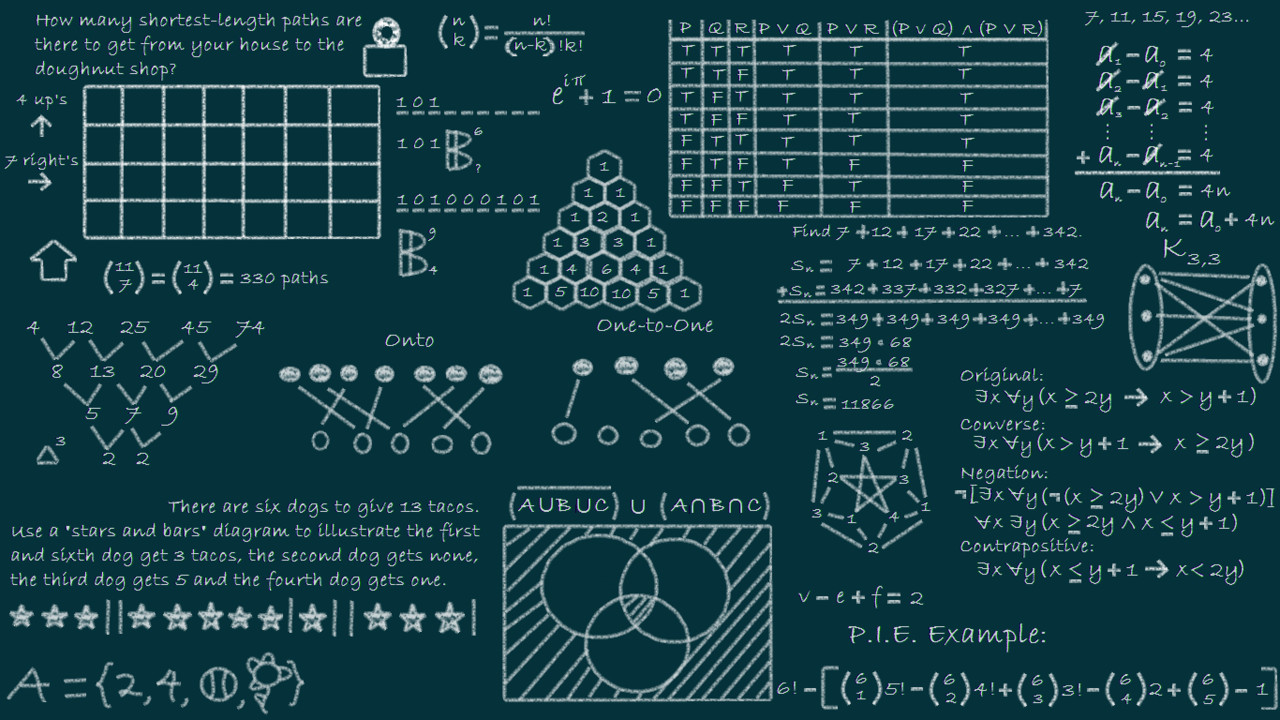
Categories:
7 minute read
In computer science, algorithm design is a critical area that helps solve complex problems efficiently. Among the many techniques used to develop efficient algorithms, Divide-and-Conquer stands out as one of the most powerful and widely-used methods. This approach breaks down large, complex problems into smaller, more manageable subproblems, solves them recursively, and then combines the solutions to solve the original problem. From sorting algorithms like Merge Sort and Quick Sort to solving mathematical problems, the divide-and-conquer method is central to modern computing.
In this blog post, we will explore the divide-and-conquer method in detail, how it works, its advantages, common applications, and examples of algorithms that rely on this strategy.
What is the Divide-and-Conquer Method?
Divide-and-conquer is an algorithm design paradigm based on breaking a problem down into smaller subproblems, solving each subproblem independently, and combining their solutions to solve the original problem. The basic structure of a divide-and-conquer algorithm follows three steps:
Divide: Split the problem into smaller subproblems.
Conquer: Solve each subproblem recursively. If the subproblem is small enough, solve it directly.
Combine: Merge the solutions of the subproblems to solve the original problem. By dividing the problem into smaller pieces, this method often reduces the complexity and allows for more efficient algorithms.
Key Characteristics of Divide-and-Conquer
Recursion: Divide-and-conquer algorithms often use recursion to break down the problem and solve the smaller instances. This recursive nature makes the method very powerful but sometimes challenging to implement.
Subproblem Independence: The subproblems must be independent of each other. This independence ensures that solving one subproblem does not affect the solution to another.
Combining the Results: After solving the subproblems, the results must be merged to form the final solution. This step can sometimes be computationally expensive, depending on the algorithm.
How Divide-and-Conquer Works
To understand the divide-and-conquer strategy, let’s break down its process with a generic example.
Imagine you are given a problem ( P ) that is too large or complex to solve directly. Instead of trying to solve ( P ) as a whole, you first divide ( P ) into smaller subproblems ( P_1 ), ( P_2 ), ( P_3 ), and so on. Each of these subproblems is easier to solve compared to the original problem. You solve each subproblem individually, possibly using the same divide-and-conquer approach if the subproblem is still large. Once you have the solutions for the subproblems, you combine them to get the final solution for the original problem ( P ).
Example of Merge Sort
One of the classic examples of the divide-and-conquer method is the Merge Sort algorithm. Let’s break down how it applies the divide-and-conquer approach:
Divide: Split the array into two halves.
Conquer: Recursively sort each half of the array.
Combine: Merge the two sorted halves back together to form the sorted array. Let’s say you have an unsorted array of numbers: [38, 27, 43, 3, 9, 82, 10]. Here’s how Merge Sort works:
Step 1 (Divide): Split the array into two halves: [38, 27, 43, 3] and [9, 82, 10].
Step 2 (Conquer): Recursively apply merge sort to each half. Split them further until you have arrays with one element:
[38], [27], [43], [3], [9], [82], [10].
Step 3 (Combine): Merge the sorted subarrays:
Merge [38] and [27] → [27, 38].
Merge [43] and [3] → [3, 43].
Merge [9] and [82] → [9, 82].
Merge [27, 38] and [3, 43] → [3, 27, 38, 43].
Merge [9, 82] and [10] → [9, 10, 82].
Finally, merge [3, 27, 38, 43] and [9, 10, 82] → [3, 9, 10, 27, 38, 43, 82]. By recursively dividing the array and sorting smaller subarrays, Merge Sort efficiently sorts the entire list in O(n log n) time complexity.
Advantages of the Divide-and-Conquer Approach
The divide-and-conquer method offers several benefits that make it an ideal choice for solving complex problems in computer algorithms:
Efficiency: Many divide-and-conquer algorithms, like Merge Sort and Quick Sort, are much more efficient than straightforward iterative methods for large datasets. The time complexity for these algorithms is often O(n log n), significantly better than O(n^2) algorithms like Bubble Sort.
Parallelism: The independence of subproblems makes divide-and-conquer algorithms ideal for parallel processing. Since each subproblem can be solved independently, the work can be distributed across multiple processors, making the algorithm faster when parallel computing resources are available.
Simplified Problem-Solving: Dividing a complex problem into smaller parts often simplifies the problem-solving process. This approach allows for recursive solutions, which are more intuitive for certain types of problems, like tree traversals or sorting.
Reusability: The divide-and-conquer technique is versatile and can be reused across different domains, from sorting and searching to optimization problems and mathematical computations. Once you understand how to apply the method, you can adapt it to a wide variety of problems.
Common Applications of Divide-and-Conquer Algorithms
- Sorting Algorithms
As discussed earlier, sorting algorithms like Merge Sort and Quick Sort rely heavily on the divide-and-conquer approach. Merge Sort divides the array into smaller arrays and merges the sorted subarrays, while Quick Sort partitions the array based on a pivot element and recursively sorts the subarrays.
- Binary Search
Another classic example of divide-and-conquer is Binary Search, which is used to find an element in a sorted array. The array is divided into two halves, and the algorithm determines which half to search based on a comparison with the middle element. The process repeats until the element is found or the search space is reduced to zero.
- Matrix Multiplication
The divide-and-conquer technique can also be used for matrix multiplication, particularly for large matrices. Strassen’s Algorithm is a well-known divide-and-conquer method for multiplying two matrices that is more efficient than the conventional approach. By splitting the matrices into smaller submatrices, Strassen’s algorithm reduces the number of multiplications required, making the process faster.
- Closest Pair of Points Problem
In computational geometry, the Closest Pair of Points problem is a great example of the divide-and-conquer method in action. Given a set of points on a plane, the goal is to find the pair of points that are closest together. The problem is solved by dividing the points into two halves, solving the problem recursively for each half, and then merging the solutions while checking for the closest pair across the dividing line.
- Dynamic Programming
Dynamic programming often borrows concepts from divide-and-conquer. Problems like Longest Common Subsequence or Matrix Chain Multiplication are solved by breaking down the original problem into overlapping subproblems and combining their solutions to optimize the result.
Challenges and Limitations
While the divide-and-conquer method is powerful, it is not without its challenges:
Recursive Overhead: Recursive algorithms often require more memory due to the need to maintain multiple function calls on the stack. For large inputs, this can lead to stack overflow errors if the recursion depth exceeds the system’s capacity.
Combining Step Complexity: In some cases, the process of combining the solutions from the subproblems can be computationally expensive. For example, in Merge Sort, merging two sorted arrays requires time proportional to the size of the arrays, adding an extra layer of complexity to the overall algorithm.
Subproblem Size: The efficiency of the divide-and-conquer method depends on the size of the subproblems. If the subproblems are not significantly smaller than the original problem, the method may not offer significant performance improvements.
Conclusion
The divide-and-conquer method is a powerful algorithmic paradigm that has widespread applications in computer science. From sorting and searching to optimization and computational geometry, this approach helps solve complex problems efficiently by breaking them down into manageable subproblems. While it comes with some challenges, such as recursive overhead and combining step complexity, its advantages in terms of efficiency, parallelism, and simplicity make it an essential technique for algorithm designers.
Understanding the divide-and-conquer method not only helps in mastering important algorithms like Merge Sort and Quick Sort but also provides a framework for solving a broad range of problems across various domains. If you’re looking to improve your problem-solving skills in algorithm design, mastering divide-and-conquer is a great place to start.