Do-while Loops in Kotlin
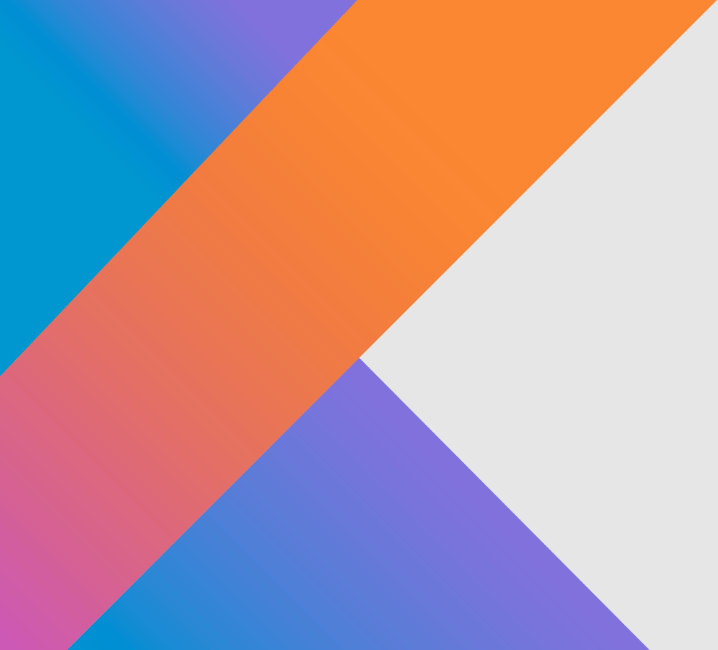
Categories:
5 minute read
Kotlin, as a modern programming language, provides several control flow structures to help developers write efficient and readable code. Among these structures, the do-while loop stands out as a unique iteration mechanism that ensures at least one execution of a code block before checking the loop condition. In this comprehensive guide, we’ll explore the do-while loop in Kotlin, its syntax, use cases, best practices, and common pitfalls to avoid.
What is a Do-while Loop?
A do-while loop is a control flow statement that executes a block of code at least once before checking the condition for subsequent iterations. This behavior distinguishes it from its cousin, the while loop, which evaluates the condition before executing the code block. The fundamental structure looks like this:
do {
// Code block to be executed
} while (condition)
Key Characteristics of Do-while Loops
1. Guaranteed First Execution
The most distinctive feature of a do-while loop is that it guarantees at least one execution of the code block. This makes it particularly useful when you need to perform an action before knowing whether to continue with additional iterations.
var userInput: String
do {
println("Please enter a positive number (or 'quit' to exit):")
userInput = readLine() ?: ""
// Process the input here
} while (userInput != "quit")
2. Condition Evaluation at the End
Unlike while loops, do-while loops evaluate their condition after executing the code block. This timing difference can significantly impact how you structure your code and handle initialization of variables used in the condition.
var counter = 0
do {
counter++
println("Counter value: $counter")
} while (counter < 5)
Common Use Cases
1. Input Validation
One of the most practical applications of do-while loops is input validation. When you need to ensure that user input meets certain criteria, a do-while loop can repeatedly prompt for input until valid data is received.
fun getValidAge(): Int {
var age: Int
do {
println("Enter your age (must be between 0 and 120):")
age = readLine()?.toIntOrNull() ?: -1
} while (age < 0 || age > 120)
return age
}
2. Menu-Driven Programs
Do-while loops are excellent for implementing menu-driven programs where you want to display options and process user choices repeatedly until a specific exit condition is met.
fun showMenu() {
var choice: Int
do {
println("\n1. Add new item")
println("2. View all items")
println("3. Delete item")
println("4. Exit")
println("\nEnter your choice (1-4):")
choice = readLine()?.toIntOrNull() ?: 0
when (choice) {
1 -> addItem()
2 -> viewItems()
3 -> deleteItem()
4 -> println("Exiting program...")
else -> println("Invalid choice! Please try again.")
}
} while (choice != 4)
}
3. Processing Data Streams
When working with data streams or iterators, do-while loops can be useful for processing elements when you know there’s at least one item to process.
fun processDataStream(iterator: Iterator<String>) {
do {
val item = iterator.next()
processItem(item)
} while (iterator.hasNext())
}
Best Practices and Optimization
1. Keep the Loop Body Focused
Maintain a single responsibility within your do-while loop. If the loop body becomes too complex, consider breaking it down into smaller functions:
do {
val input = getUserInput()
val isValid = validateInput(input)
if (isValid) {
processInput(input)
}
} while (!isValid)
2. Guard Against Infinite Loops
Always ensure there’s a way to exit the loop. Include proper condition updates and error handling:
var retryCount = 0
val maxRetries = 3
do {
try {
// Attempt operation
break // Exit loop on success
} catch (e: Exception) {
retryCount++
println("Attempt $retryCount failed")
}
} while (retryCount < maxRetries)
3. Consider Performance Impact
For performance-critical applications, be mindful of the condition evaluation cost. If possible, cache complex condition results:
do {
val result = performExpensiveOperation()
val shouldContinue = evaluateResult(result)
} while (shouldContinue)
Common Pitfalls to Avoid
1. Forgetting Break Conditions
One of the most common mistakes is forgetting to include proper break conditions, leading to infinite loops:
// Problematic code
do {
processData()
// Missing condition update or break statement
} while (true)
// Better approach
var isProcessing = true
do {
val result = processData()
isProcessing = result.needsMoreProcessing()
} while (isProcessing)
2. Incorrect Variable Scope
Be careful with variable scope in do-while loops, especially when the condition depends on variables declared inside the loop:
// Incorrect scope
do {
val input = readLine()
} while (input != null) // Error: input not accessible here
// Correct scope
var input: String?
do {
input = readLine()
} while (input != null)
3. Overcomplicating Loop Conditions
Keep loop conditions simple and readable. Complex conditions can lead to maintenance issues and bugs:
// Overly complex condition
do {
// Process data
} while (condition1 && (condition2 || condition3) && !condition4)
// Better approach: Break down complex conditions
do {
// Process data
val shouldContinue = evaluateComplexConditions(condition1, condition2, condition3, condition4)
} while (shouldContinue)
Conclusion
Do-while loops in Kotlin offer a powerful way to handle iterations where at least one execution is required. They excel in scenarios involving user input, menu-driven programs, and data processing. By following best practices and avoiding common pitfalls, you can effectively utilize do-while loops to write more robust and maintainable code.
Remember that while do-while loops are valuable tools in your programming arsenal, they should be used judiciously. Always consider whether a do-while loop is the most appropriate solution for your specific use case, and don’t hesitate to explore alternative control flow structures when they might better serve your needs.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.