Function Types in Kotlin
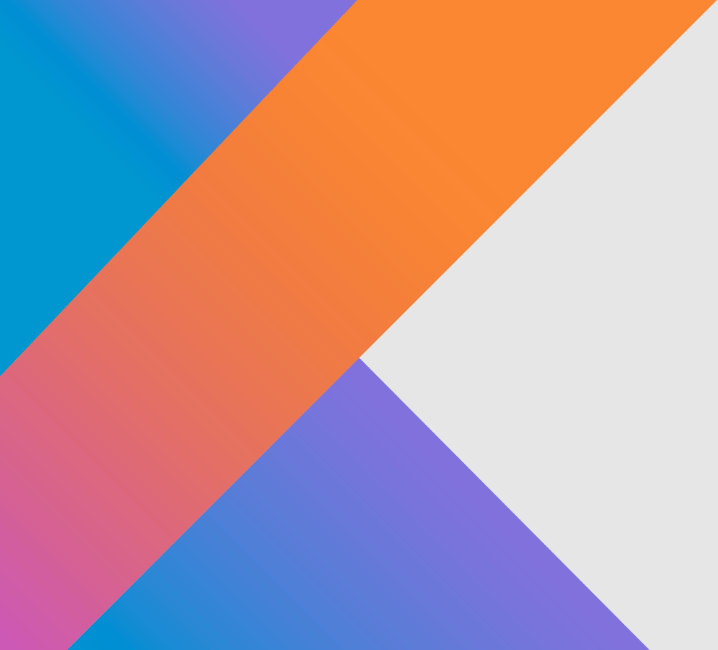
Categories:
4 minute read
Kotlin, a modern and expressive programming language, provides powerful features for handling functions. One of the most versatile aspects of Kotlin is its support for function types, which allow developers to treat functions as first-class citizens. This means functions can be assigned to variables, passed as arguments, and returned from other functions. Understanding function types is crucial for writing clean, concise, and functional Kotlin code.
In this blog post, we will explore Kotlin’s function types, their syntax, and use cases, covering everything from basic function types to higher-order functions and lambda expressions.
What Are Function Types?
Function types in Kotlin describe the type signature of functions, allowing them to be treated as values. A function type specifies the input parameters and the return type of a function.
Syntax of Function Types
The general syntax of a function type in Kotlin is:
(parameterType1, parameterType2, ...) -> ReturnType
For example, consider a function that takes two integers and returns their sum:
val sum: (Int, Int) -> Int = { a, b -> a + b }
Here:
(Int, Int) -> Int
represents the function type, meaning it takes twoInt
values and returns anInt
.{ a, b -> a + b }
is a lambda expression assigned to the variablesum
.
Common Function Types
Kotlin provides several function types that can be used in different contexts. Let’s explore some of the most common ones.
1. Function Types with Multiple Parameters
A function that takes multiple arguments and returns a result can be defined as:
val multiply: (Int, Int) -> Int = { x, y -> x * y }
println(multiply(4, 5)) // Output: 20
2. Function Types with No Parameters
A function type that takes no parameters can be written as:
val greet: () -> String = { "Hello, Kotlin!" }
println(greet()) // Output: Hello, Kotlin!
3. Function Types with No Return Value (Unit)
If a function does not return a meaningful value, it returns Unit
(similar to void
in Java):
val printMessage: (String) -> Unit = { message -> println(message) }
printMessage("This is Kotlin!") // Output: This is Kotlin!
4. Nullable Function Types
Function types can be nullable, meaning the function reference can be null
:
var nullableFunction: ((Int, Int) -> Int)? = null
nullableFunction = { a, b -> a + b }
println(nullableFunction?.invoke(3, 4)) // Output: 7
Higher-Order Functions
Higher-order functions are functions that accept other functions as parameters or return functions as results. These are widely used in Kotlin for functional programming.
Example of a Higher-Order Function
fun operateOnNumbers(a: Int, b: Int, operation: (Int, Int) -> Int): Int {
return operation(a, b)
}
val sum = operateOnNumbers(5, 10) { x, y -> x + y }
println(sum) // Output: 15
In this example, operateOnNumbers
is a higher-order function that takes an operation as a function parameter and applies it to a
and b
.
Lambda Expressions and Anonymous Functions
Lambda expressions and anonymous functions are used to define function literals in Kotlin. These function literals can be stored in variables and passed around as parameters.
Lambda Expressions
A lambda expression is an unnamed function that can be assigned to a variable:
val square: (Int) -> Int = { number -> number * number }
println(square(6)) // Output: 36
Anonymous Functions
An anonymous function is similar to a lambda but allows specifying the return type explicitly:
val subtract = fun(x: Int, y: Int): Int { return x - y }
println(subtract(10, 3)) // Output: 7
Inline Functions for Performance Optimization
Kotlin provides inline functions to reduce overhead when using higher-order functions by inlining the function body at the call site.
Example of an Inline Function
inline fun execute(action: () -> Unit) {
action()
}
execute { println("This function is inlined!") }
Inlining helps avoid extra object creation and improves performance, especially when working with lambda expressions.
Conclusion
Function types in Kotlin make it easier to work with higher-order functions, lambda expressions, and functional programming paradigms. Understanding function types helps developers write more concise, readable, and efficient code.
Key Takeaways
- Function types describe the input parameters and return type of a function.
- Functions can be stored in variables and passed as arguments.
- Higher-order functions accept functions as parameters or return them as results.
- Lambda expressions and anonymous functions provide flexible ways to define function literals.
- Inline functions help optimize performance by eliminating function call overhead.
By mastering function types, you can unlock the full potential of Kotlin’s expressive and functional capabilities, making your code more elegant and maintainable.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.