Hardware Description Languages: Verilog Basics
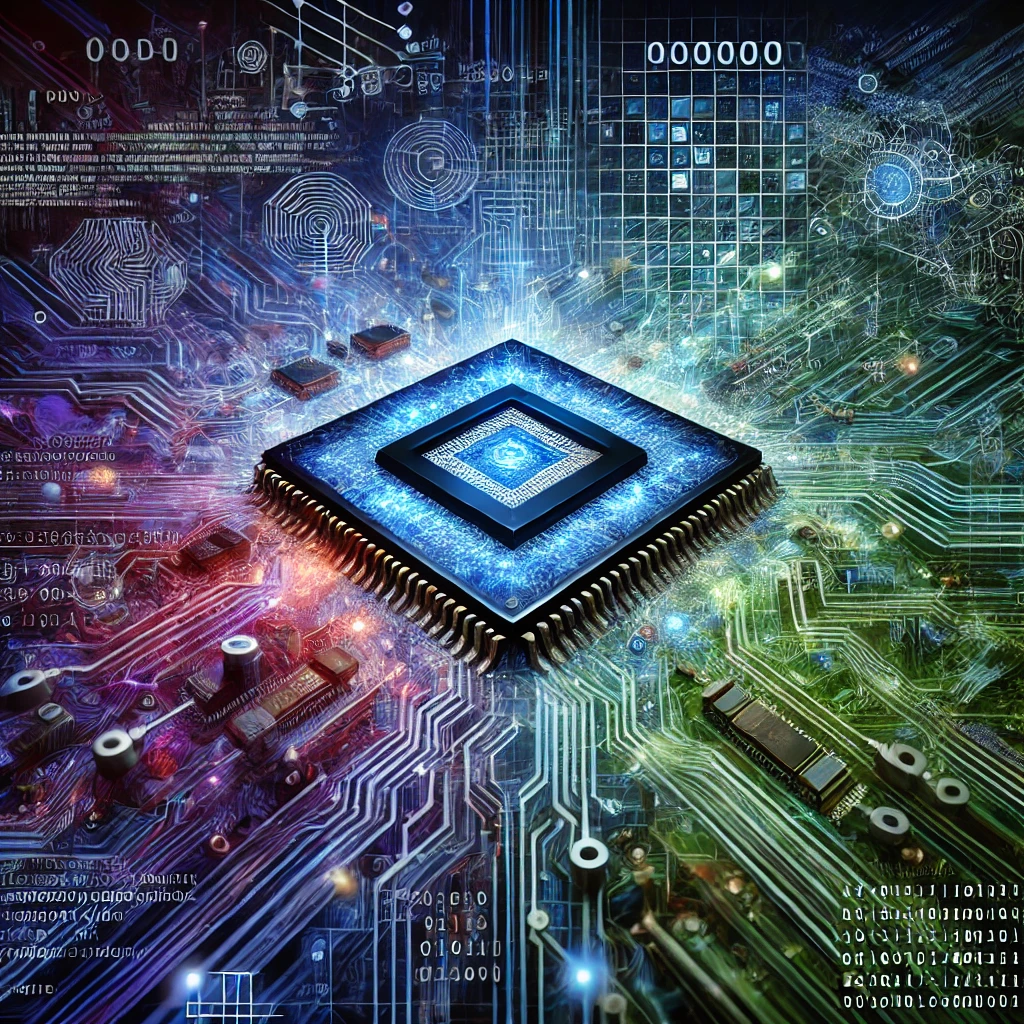
Categories:
6 minute read
In the world of digital design and electronic engineering, Hardware Description Languages (HDLs) play a crucial role in describing and modeling complex digital systems. Among these languages, Verilog stands out as one of the most widely used and powerful tools for designing and simulating digital circuits. In this blog post, we’ll delve into the basics of Verilog, exploring its fundamental concepts, syntax, and applications.
What is Verilog?
Verilog, short for “Verification Logic,” is a hardware description language used to model electronic systems. It was originally developed by Phil Moorby at Gateway Design Automation in 1984 and later became an IEEE standard in 1995. Verilog allows engineers to describe the behavior and structure of digital circuits and systems at various levels of abstraction, from high-level behavioral descriptions to low-level gate-level implementations.
Why Use Verilog?
There are several compelling reasons to use Verilog in digital design:
Abstraction: Verilog allows designers to work at different levels of abstraction, from system-level behavior down to gate-level implementation.
Simulation: Verilog designs can be simulated before actual hardware implementation, saving time and resources.
Synthesis: Verilog code can be synthesized into actual hardware designs for FPGAs or ASICs.
Standardization: As an IEEE standard, Verilog is widely supported by various tools and platforms in the industry.
Modularity: Verilog supports hierarchical design, allowing complex systems to be broken down into manageable modules. Now that we understand the importance of Verilog, let’s dive into its basic concepts and syntax.
Verilog Basics
Modules
The fundamental building block in Verilog is the module. A module is a self-contained unit that represents a component of a digital system. It can be as simple as a single logic gate or as complex as an entire microprocessor. Here’s the basic structure of a Verilog module:
module module_name(port_list);
// Port declarations
// Internal signal declarations
// Behavioral or structural description
endmodule```
Data Types
Verilog supports several data types to represent different kinds of signals and variables:
* **Wire**: Represents a physical connection between components. It doesn't store a value.
* **Reg**: Represents a variable that can store a value.
* **Integer**: A 32-bit signed integer.
* **Real**: A double-precision floating-point number.
* **Time**: Used for simulation timekeeping.
Here's an example of how to declare these data types:
```bash
wire w;
reg r;
integer i;
real x;
time t;
Value Set
Verilog uses a four-value system to represent logic levels:
0: Logic zero, false
1: Logic one, true
x: Unknown logic value
z: High impedance state Operators
Verilog supports a wide range of operators, including:
Arithmetic operators: +, -, *, /, %
Logical operators: &&, ||, !
Relational operators: <, >, <=, >=, ==, !=
Bitwise operators: &, |, ^, ~
Reduction operators: &, ~&, |, ~|, ^, ~^
Shift operators: «, »
Concatenation operator: {}
Conditional operator: ?: Behavioral Modeling
Behavioral modeling in Verilog allows designers to describe the functionality of a circuit without specifying its exact structure. This is typically done using procedural blocks like initial
and always
.
The initial
block is executed only once at the beginning of simulation:
initial begin
// Initialization code
end
The always
block is used for describing continuous behavior:
always @(posedge clk) begin
// Sequential logic
end
Structural Modeling
Structural modeling involves describing a circuit in terms of its components and their interconnections. This is done using module instantiation and continuous assignments.
Module instantiation:
module_name instance_name (
.port1(signal1),
.port2(signal2)
);
Continuous assignment:
assign output_wire = input1 & input2;
A Simple Example: 4-bit Adder
Let’s put these concepts together by designing a simple 4-bit adder:
module adder_4bit(
input [3:0] a,
input [3:0] b,
input cin,
output [3:0] sum,
output cout
);
wire [4:0] temp;
assign temp = a + b + cin;
assign sum = temp[3:0];
assign cout = temp[4];
endmodule```
In this example, we've created a module called `adder_4bit` with inputs `a`, `b`, and `cin` (carry-in), and outputs `sum` and `cout` (carry-out). The addition is performed using a continuous assignment, and the result is split into the sum and carry-out.
## Testbenches
An essential aspect of Verilog design is verification through simulation. This is typically done using testbenches. A testbench is a Verilog module that instantiates the design under test (DUT) and provides stimulus to verify its functionality.
Here's a simple testbench for our 4-bit adder:
```bash
module adder_4bit_tb;
reg [3:0] a, b;
reg cin;
wire [3:0] sum;
wire cout;
// Instantiate the DUT
adder_4bit dut(
.a(a),
.b(b),
.cin(cin),
.sum(sum),
.cout(cout)
);
// Stimulus
initial begin
$monitor("Time=%0t a=%b b=%b cin=%b sum=%b cout=%b",
$time, a, b, cin, sum, cout);
a = 4'b0000; b = 4'b0000; cin = 0; #10;
a = 4'b0001; b = 4'b0001; cin = 0; #10;
a = 4'b1111; b = 4'b0001; cin = 0; #10;
a = 4'b1111; b = 4'b0001; cin = 1; #10;
$finish;
end
endmodule```
This testbench creates instances of the input signals, instantiates the 4-bit adder, and then applies various test vectors to verify its functionality.
## Advanced Verilog Concepts
While we've covered the basics, Verilog offers many more advanced features for complex digital design:
* **Tasks and Functions**: These allow you to create reusable code blocks within modules.
* **Parameterized Modules**: Enable the creation of flexible, scalable designs.
* **Generate Statements**: Useful for creating repetitive structures in your design.
* **System Tasks and Functions**: Provide additional functionality for simulation and debugging (e.g., `$display`, `$monitor`, `$finish`).
* **Timing Controls**: Allow for the specification of delays in your design.
* **Interfaces**: Simplify the connections between modules in complex designs.
* **Assertions**: Help in verifying design properties and catching bugs early in the design process.
## Conclusion
Verilog is a powerful and versatile hardware description language that forms the backbone of modern digital design. From simple logic gates to complex microprocessors, Verilog provides the tools needed to describe, simulate, and synthesize digital circuits.
In this post, we've scratched the surface of what Verilog can do, covering its basic syntax, data types, modeling techniques, and a simple example of a 4-bit adder. However, mastering Verilog requires practice and exposure to more complex designs.
As you continue your journey in digital design, you'll find that Verilog, along with its counterpart VHDL, opens up a world of possibilities in creating efficient, scalable, and robust digital systems. Whether you're designing for FPGAs, ASICs, or just exploring the world of digital logic, Verilog provides a solid foundation for turning your digital designs into reality.
Remember, the key to becoming proficient in Verilog is practice. Start with simple designs, use simulation to verify your work, and gradually tackle more complex projects. Happy coding!