Jump Expressions in Kotlin
break
, continue
, and return
expressions in Kotlin.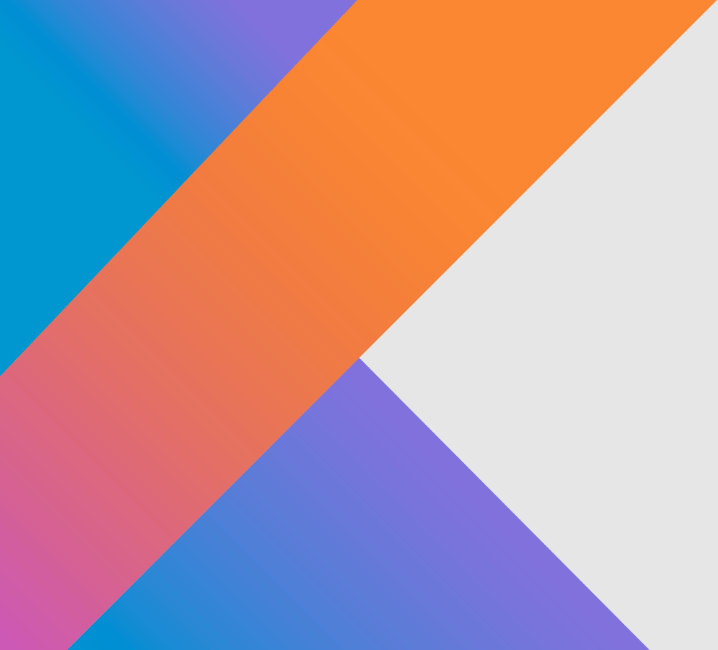
Categories:
4 minute read
Introduction
Kotlin, a modern and expressive programming language developed by JetBrains, provides several control flow mechanisms that enhance readability and efficiency. Among these are jump expressions, which control the flow of execution in loops and functions. The primary jump expressions in Kotlin are:
break
– Exits the nearest enclosing loop.continue
– Skips the current iteration of a loop and moves to the next.return
– Exits a function and optionally returns a value.
Jump expressions allow developers to control how loops and functions execute, making code more readable and efficient. In this blog post, we will explore break, continue, and return in detail, their syntax, use cases, and best practices, with practical examples.
1. The break
Expression
What is break
?
The break
expression is used to exit the nearest enclosing loop immediately. When break
is encountered, the loop terminates, and control moves to the next statement outside the loop.
Syntax:
break
Using break
in a Loop
fun main() {
for (i in 1..10) {
if (i == 5) {
println("Breaking at $i")
break
}
println("Iteration: $i")
}
println("Loop exited.")
}
Output:
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Breaking at 5
Loop exited.
Using break
with Nested Loops (Labeled Breaks)
Kotlin allows labeled breaks to exit from specific loops in nested loops.
fun main() {
outer@ for (i in 1..3) {
for (j in 1..3) {
if (j == 2) break@outer
println("i=$i, j=$j")
}
}
println("Exited outer loop.")
}
Output:
i=1, j=1
Exited outer loop.
2. The continue
Expression
What is continue
?
The continue
expression is used to skip the current iteration of a loop and move directly to the next iteration.
Syntax:
continue
Using continue
in a Loop
fun main() {
for (i in 1..5) {
if (i == 3) {
println("Skipping iteration: $i")
continue
}
println("Iteration: $i")
}
}
Output:
Iteration: 1
Iteration: 2
Skipping iteration: 3
Iteration: 4
Iteration: 5
Using continue
with Labeled Loops
fun main() {
outer@ for (i in 1..3) {
for (j in 1..3) {
if (j == 2) continue@outer
println("i=$i, j=$j")
}
}
}
Output:
i=1, j=1
i=2, j=1
i=3, j=1
3. The return
Expression
What is return
?
The return
expression is used to exit a function and optionally return a value.
Syntax:
return
return value
Using return
in Functions
fun greet(name: String): String {
return "Hello, $name!"
}
fun main() {
println(greet("Alice"))
}
Output:
Hello, Alice!
Returning from a Loop in a Function
fun findEven(numbers: List<Int>): Int? {
for (num in numbers) {
if (num % 2 == 0) return num
}
return null
}
fun main() {
val numbers = listOf(1, 3, 7, 8, 9)
println("First even number: ${findEven(numbers)}")
}
Output:
First even number: 8
Returning from a Lambda (Labeled Return)
By default, return
inside a lambda expression exits the entire function. To return only from the lambda, use a labeled return.
fun main() {
listOf(1, 2, 3, 4).forEach {
if (it == 3) return@forEach
println(it)
}
println("Loop finished")
}
Output:
1
2
4
Loop finished
Best Practices for Using Jump Expressions
- Use
break
only when necessary – Avoid excessive use, as it may lead to unexpected behavior in loops. - Prefer
continue
over complex conditionals – Instead of deeply nestedif
statements, usecontinue
to skip iterations. - Be cautious with
return
in lambdas – Unlabeledreturn
inside a lambda will exit the enclosing function. - Use labeled breaks wisely – While useful, overusing labels can reduce readability.
- Consider using higher-order functions – In many cases, functions like
filter
andmap
can eliminate the need for jump expressions.
Conclusion
Jump expressions in Kotlin (break
, continue
, and return
) provide powerful control over loops and functions. They help streamline the flow of execution, making code more efficient and readable. By understanding when and how to use these expressions effectively, you can write cleaner and more maintainable Kotlin programs.
Whether you are controlling loops with break
and continue
or managing function exits with return
, mastering these expressions will significantly enhance your Kotlin programming skills.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.