Launching Coroutines in Kotlin
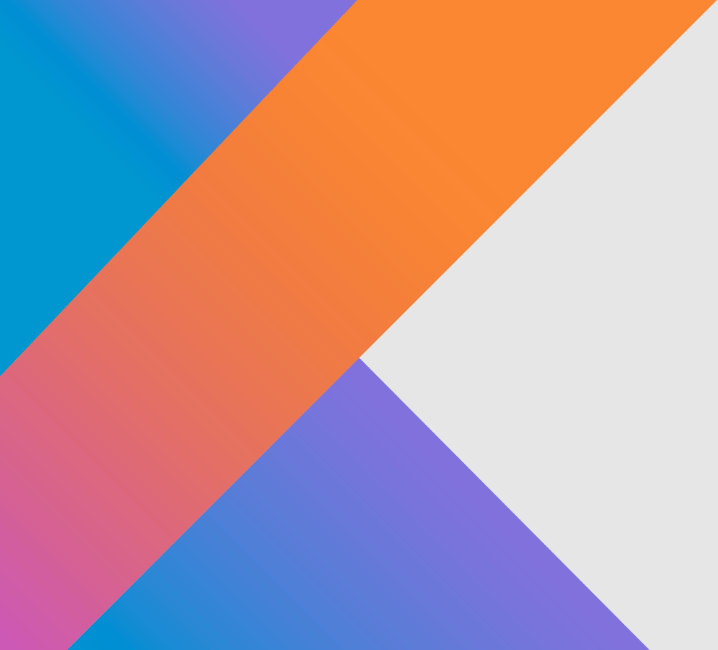
Categories:
5 minute read
Kotlin coroutines have revolutionized asynchronous programming, offering a more structured and concise way to handle concurrency. Unlike traditional multithreading, coroutines provide an efficient and lightweight alternative, making it easier to manage background tasks without blocking the main thread.
In this blog post, we’ll explore how to launch coroutines in Kotlin, the different coroutine builders available, and best practices for using them effectively. By the end, you’ll have a solid understanding of how to work with coroutines and improve the performance of your Kotlin applications.
What Are Coroutines in Kotlin?
Coroutines in Kotlin are a concurrency design pattern that allows developers to write asynchronous code in a sequential style. They help manage tasks that would otherwise require callbacks or explicit thread management.
A coroutine is like a lightweight thread that can be suspended and resumed without blocking the underlying thread. This makes it more efficient than traditional threading mechanisms, as coroutines use fewer resources while achieving the same result.
Key Features of Coroutines:
- Lightweight: Coroutines don’t require new threads; instead, they use existing threads efficiently.
- Non-blocking: They enable asynchronous execution without blocking the main thread.
- Structured concurrency: Kotlin provides built-in coroutine scopes and job hierarchies to manage lifecycle easily.
- Seamless integration: Coroutines work well with existing Kotlin features, such as suspending functions and flow.
Now that we understand what coroutines are, let’s dive into how to launch them in Kotlin.
How to Launch Coroutines in Kotlin
To start a coroutine in Kotlin, we use coroutine builders. These builders determine the lifecycle and execution behavior of coroutines.
1. Using launch
The launch
builder is used to start a coroutine that runs in the background and doesn’t return a result. It’s commonly used for fire-and-forget tasks like updating UI components or performing I/O operations.
Example: Launching a Simple Coroutine
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
println("Coroutine started!")
delay(1000)
println("Coroutine completed!")
}
println("Main function continues...")
}
How It Works:
runBlocking
is used to keep the main thread alive until coroutines complete.launch
starts a new coroutine.delay(1000)
suspends the coroutine for one second without blocking the main thread.- The “Main function continues…” line executes immediately since
launch
doesn’t block execution.
2. Using async
The async
builder is used when we need a coroutine to return a result. It returns a Deferred object, which can be awaited using await()
.
Example: Using async
to Perform Concurrent Tasks
import kotlinx.coroutines.*
fun main() = runBlocking {
val result = async {
delay(1000)
42
}
println("Waiting for result...")
println("Result: ${result.await()}")
}
Key Takeaways:
async
is used when we need a result.- The coroutine runs asynchronously but returns a Deferred object.
- Calling
await()
suspends execution until the coroutine completes and returns the result.
3. Using runBlocking
The runBlocking
builder blocks the current thread until all coroutines inside it complete. It’s mainly used for quick tests or to bridge between synchronous and asynchronous code.
Example: Blocking the Main Thread
import kotlinx.coroutines.*
fun main() {
runBlocking {
println("Starting runBlocking")
launch {
delay(1000)
println("Inside coroutine")
}
println("End of runBlocking")
}
}
Why Use runBlocking
?
- It ensures that the program doesn’t terminate before coroutines complete.
- Useful in main functions or unit tests where coroutines need to complete execution.
Choosing the Right Coroutine Builder
Coroutine Builder | Returns | Blocking Behavior | Use Case |
---|---|---|---|
launch | Job | Non-blocking | Fire-and-forget tasks |
async | Deferred | Non-blocking | When a result is needed |
runBlocking | None | Blocks thread | Bridging synchronous and asynchronous code |
Coroutine Scopes and Contexts
1. CoroutineScope
Coroutines need a scope to manage their lifecycle. The most commonly used scopes are:
GlobalScope
: Creates coroutines that live as long as the entire application. Not recommended for structured concurrency.CoroutineScope
: Provides a structured way to launch coroutines and manage their lifecycle.viewModelScope
(Android-specific): Used for coroutines withinViewModel
instances.lifecycleScope
(Android-specific): Tied to an Android component’s lifecycle (likeActivity
orFragment
).
Example: Using CoroutineScope
class MyClass {
private val coroutineScope = CoroutineScope(Dispatchers.Default)
fun doWork() {
coroutineScope.launch {
println("Working in coroutine scope")
}
}
}
2. Dispatchers: Controlling Coroutine Execution Context
Coroutines run on specific dispatchers, which determine the thread they execute on.
Dispatcher | Description |
---|---|
Dispatchers.Default | Optimized for CPU-intensive tasks. |
Dispatchers.IO | Optimized for network and database operations. |
Dispatchers.Main | Used for UI updates (Android). |
Dispatchers.Unconfined | Runs coroutine in the calling thread until suspension. |
Example: Using Different Dispatchers
fun main() = runBlocking {
launch(Dispatchers.Default) {
println("Running on Default Dispatcher")
}
launch(Dispatchers.IO) {
println("Running on IO Dispatcher")
}
}
Best Practices for Using Coroutines
- Use structured concurrency: Avoid using
GlobalScope
and preferCoroutineScope
for better lifecycle management. - Choose the right dispatcher: Use
Dispatchers.IO
for I/O operations andDispatchers.Default
for CPU-intensive tasks. - Handle exceptions: Use
try-catch
blocks or structured exception handling withCoroutineExceptionHandler
. - Cancel unnecessary coroutines: Use
Job.cancel()
orwithTimeout()
to prevent memory leaks. - Avoid blocking the main thread: Use
delay()
instead ofThread.sleep()
for suspending execution.
Conclusion
Kotlin coroutines provide a powerful yet simple way to handle asynchronous programming. By understanding the different coroutine builders (launch
, async
, runBlocking
), coroutine scopes, and dispatchers, developers can write efficient and maintainable concurrent applications.
By following best practices like structured concurrency and proper exception handling, you can ensure that your Kotlin applications remain performant and free from unnecessary memory leaks.
Want to learn more? Start experimenting with coroutines in your projects and see the difference they make! 🚀
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.