logical AND (&&) and OR (||) operators in C++
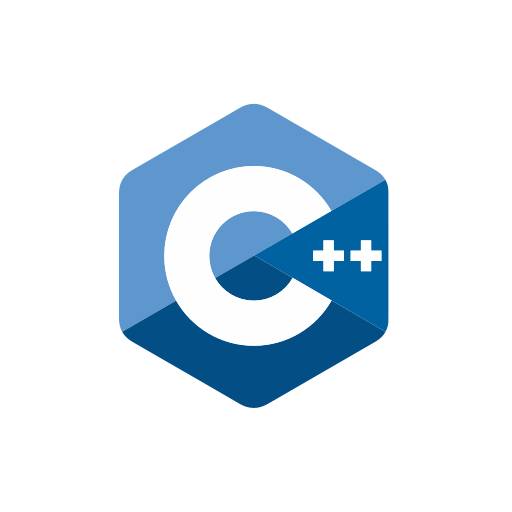
Categories:
5 minute read
The provided C++ code demonstrates the use of logical operators: AND (&&
), OR (||
), and NOT (!
), through a series of comparisons between three initialized integer variables (x
, y
, and z
).
Code
/**
* @file main.cpp
* @brief Demonstrates the use of logical AND (&&) and OR (||) operators in C++.
*
* This program initializes three integer variables, x, y, and z, and then demonstrates
* the use of logical AND (&&) and OR (||) operators by comparing these variables in
* various expressions. It also shows the use of the NOT (!) operator and explains
* the precedence of logical operators in C++.
*/
#include <iostream>
using namespace std;
int main() {
// Initialize variables
int x = 5, y = 10, z = 15;
// Display the values of x, y, and z
cout << "x = " << x << ", y = " << y << ", z = " << z << endl;
// Demonstrate logical AND (&&)
cout << "x < y && y < z = " << (x < y && y < z) << endl; // True, both conditions are true
cout << "x < y && y > z = " << (x < y && y > z) << endl; // False, second condition is false
// Demonstrate logical OR (||)
cout << "x < y || y > z = " << (x < y || y > z) << endl; // True, first condition is true
cout << "x > y || y > z = " << (x > y || y > z) << endl; // False, both conditions are false
// Demonstrate logical NOT (!)
cout << "!(x < y) = " << !(x < y) << endl; // False, negates true condition
cout << "!(x > y) = " << !(x > y) << endl; // True, negates false condition
// Explain operator precedence
cout << "priority of && is higher than ||" << endl;
// Demonstrate precedence with examples
cout << "x < y && y < z || x > z = " << (x < y && y < z || x > z) << endl;
// True, && evaluated first
cout << "x < y || y < z && x > z = " << (x < y || y < z && x > z) << endl;
// True, && evaluated first despite || appearing first
return 0;
}
Explanation
The provided C++ code demonstrates the use of logical operators: AND (&&
), OR (||
), and NOT (!
), through a series of comparisons between three initialized integer variables (x
, y
, and z
). It serves as an educational example to illustrate how these operators function in conditional statements and their precedence rules.
Initially, the code sets up three variables x
, y
, and z
with values 5, 10, and 15, respectively. This setup is crucial for the subsequent comparisons:
int x = 5, y = 10, z = 15;
The demonstration of the logical AND (&&
) operator is shown through two examples. The first example checks if x
is less than y
AND y
is less than z
, which evaluates to true since both conditions are satisfied:
cout << "x < y && y < z = " << (x < y && y < z) << endl;
The logical OR (||
) operator is similarly demonstrated. An example provided checks if x
is less than y
OR y
is greater than z
. This expression evaluates to true because the first condition is true, illustrating that only one condition needs to be true for the OR operator to result in true:
cout << "x < y || y > z = " << (x < y || y > z) << endl;
The NOT (!
) operator’s demonstration negates the truth value of the condition it precedes. For instance, negating the condition x < y
results in false because x < y
is true, and NOT true is false:
cout << "!(x < y) = " << !(x < y) << endl;
Lastly, the code touches upon the precedence of logical operators, stating that AND (&&
) has a higher precedence than OR (||
). This is crucial in understanding how complex logical expressions are evaluated. The provided examples show that even if OR appears first in an expression, the AND part is evaluated first due to its higher precedence:
cout << "x < y && y < z || x > z = " << (x < y && y < z || x > z) << endl;
This code snippet is a straightforward demonstration aimed at those familiar with C++ but perhaps not with the intricacies of logical operators and their precedence.
Output
x = 5, y = 10, z = 15
x < y && y < z = 1
x < y && y > z = 0
x < y || y > z = 1
x > y || y > z = 0
!(x < y) = 0
!(x > y) = 1
priority of && is higher than ||
x < y && y < z || x > z = 1
x < y || y < z && x > z = 1
Process finished with exit code 0```