Named Arguments in Kotlin
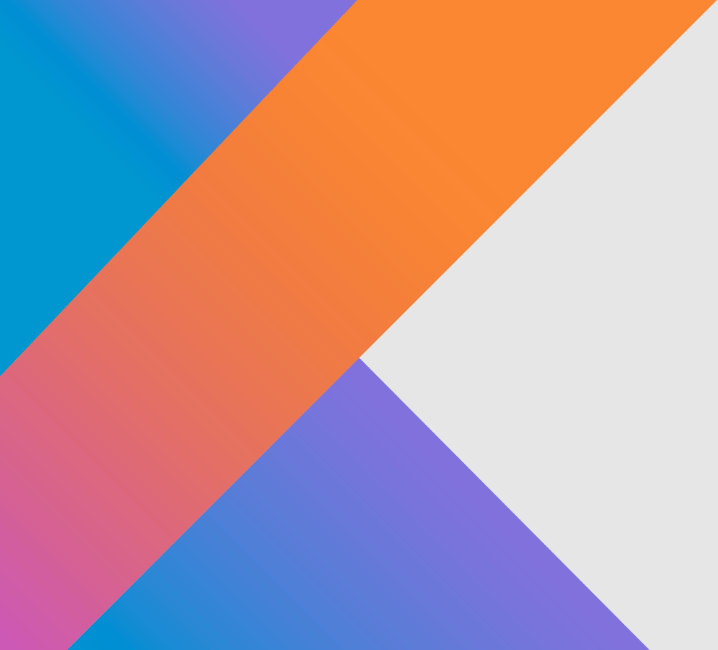
Categories:
5 minute read
Named arguments are a powerful feature in Kotlin that allows developers to specify parameter names when calling functions. This feature significantly improves code readability, maintainability, and flexibility. Let’s dive deep into how named arguments work and how to use them effectively in your Kotlin code.
Understanding Named Arguments
Named arguments allow you to explicitly specify which parameter you’re passing a value to when calling a function. Instead of relying on parameter position, you can use the parameter names directly in the function call.
Basic Syntax
Here’s how named arguments work in practice:
fun createUser(username: String, email: String, isActive: Boolean) {
// Implementation
}
// Using named arguments
createUser(
username = "john_doe",
email = "john@example.com",
isActive = true
)
Benefits of Named Arguments
Improved Code Readability
Named arguments make function calls self-documenting and easier to understand:
// Without named arguments - what do these boolean values mean?
configureServer("localhost", 8080, true, false, true)
// With named arguments - much clearer!
configureServer(
host = "localhost",
port = 8080,
enableSsl = true,
enableCompression = false,
allowAnonymous = true
)
Parameter Order Flexibility
When using named arguments, you can specify parameters in any order:
fun sendEmail(to: String, subject: String, body: String, isHtml: Boolean) {
// Implementation
}
// Parameters can be in any order when using named arguments
sendEmail(
body = "Hello, please find attached...",
isHtml = false,
to = "recipient@example.com",
subject = "Important Update"
)
Advanced Usage Patterns
Combining with Default Arguments
Named arguments work seamlessly with default arguments:
fun configureApplication(
name: String,
port: Int = 8080,
environment: String = "development",
maxThreads: Int = 10,
debug: Boolean = false
) {
// Implementation
}
// Only specify the parameters you want to customize
configureApplication(
name = "MyApp",
environment = "production",
debug = true
// port and maxThreads use default values
)
In Builder-like Functions
Named arguments can create builder-like patterns without the verbosity of traditional builders:
data class HttpRequest(
val url: String,
val method: String,
val headers: Map<String, String>,
val body: String?
)
fun createRequest(
url: String,
method: String = "GET",
headers: Map<String, String> = emptyMap(),
body: String? = null
) = HttpRequest(url, method, headers, body)
// Usage
val request = createRequest(
url = "https://api.example.com/data",
method = "POST",
headers = mapOf("Content-Type" to "application/json"),
body = """{"key": "value"}"""
)
Best Practices and Guidelines
When to Use Named Arguments
Use named arguments in these situations:
- Functions with many parameters:
fun createReport(
title: String,
startDate: LocalDate,
endDate: LocalDate,
includeCharts: Boolean = true,
exportFormat: String = "PDF",
sendEmail: Boolean = false,
recipientEmail: String? = null
) {
// Implementation
}
// Usage
createReport(
title = "Monthly Sales Report",
startDate = LocalDate.now().minusMonths(1),
endDate = LocalDate.now(),
exportFormat = "EXCEL",
sendEmail = true,
recipientEmail = "manager@example.com"
)
- Functions with multiple parameters of the same type:
fun drawRectangle(
x1: Int,
y1: Int,
x2: Int,
y2: Int,
color: String = "black"
) {
// Implementation
}
// Usage
drawRectangle(
x1 = 10,
y1 = 10,
x2 = 100,
y2 = 50,
color = "blue"
)
Mixing Named and Positional Arguments
When mixing named and positional arguments, all positional arguments must come before named ones:
fun processOrder(orderId: String, items: List<String>, discount: Double, priority: Int) {
// Implementation
}
// Valid
processOrder("ORD-123", listOf("item1", "item2"), discount = 0.1, priority = 1)
// Invalid - will not compile
// processOrder("ORD-123", items = listOf("item1", "item2"), 0.1, priority = 1)
Common Use Cases and Patterns
Configuration Functions
Named arguments are particularly useful for configuration functions:
fun configureDatabase(
host: String = "localhost",
port: Int = 5432,
database: String,
username: String,
password: String,
maxConnections: Int = 10,
timeout: Duration = Duration.ofSeconds(30),
enableSsl: Boolean = false
) {
// Implementation
}
// Usage
configureDatabase(
database = "myapp_db",
username = "admin",
password = "secret",
host = "db.example.com",
timeout = Duration.ofMinutes(1)
)
Factory Methods
Named arguments can make factory methods more expressive:
class UserProfile private constructor(
val username: String,
val email: String,
val displayName: String,
val isVerified: Boolean
) {
companion object {
fun create(
username: String,
email: String,
displayName: String = username,
isVerified: Boolean = false
) = UserProfile(
username = username,
email = email,
displayName = displayName,
isVerified = isVerified
)
}
}
// Usage
val profile = UserProfile.create(
username = "john_doe",
email = "john@example.com",
isVerified = true
)
Testing
Named arguments are valuable in test code for clarity:
@Test
fun `test user creation`() {
val user = createTestUser(
username = "test_user",
email = "test@example.com",
role = "admin",
isActive = true
)
assertThat(user).matches(
hasUsername = "test_user",
hasRole = "admin"
)
}
Conclusion
Named arguments are a powerful feature in Kotlin that significantly improves code readability and maintainability. They are particularly valuable when dealing with functions that have multiple parameters, especially when those parameters have default values or are of the same type.
Key benefits of using named arguments include:
- Enhanced code readability and self-documentation
- Flexibility in parameter order
- Reduced likelihood of parameter position errors
- Better integration with default arguments
- More expressive API design
Best practices for using named arguments:
- Use them for functions with many parameters
- Always use them when multiple parameters have the same type
- Consider them for configuration and factory methods
- Combine them with default arguments for maximum flexibility
- Use them in test code for better clarity
By following these guidelines and understanding the various use cases, you can effectively use named arguments to write more maintainable and expressive Kotlin code. Remember that while named arguments add verbosity to function calls, the benefits in terms of code clarity and safety often outweigh the extra keystrokes.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.