Nested Loops in Kotlin
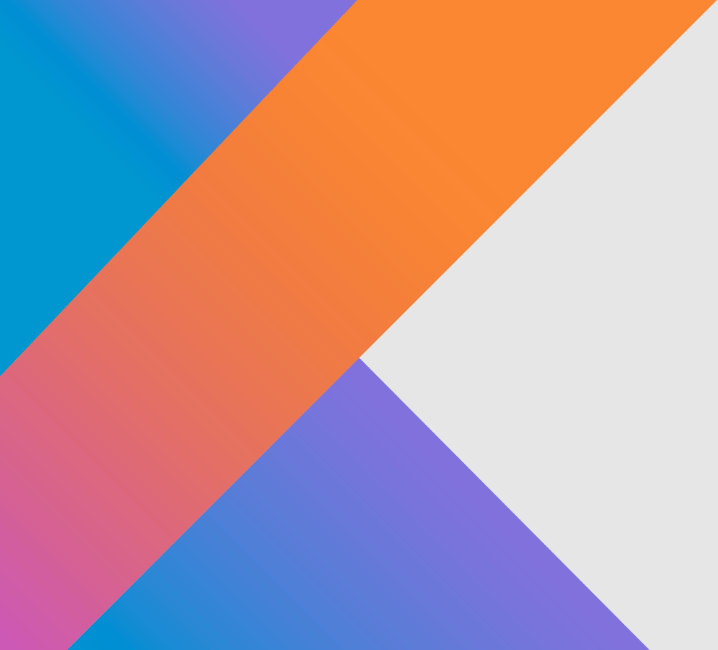
Categories:
5 minute read
Nested loops are a fundamental programming concept that allows developers to perform complex iterations and handle multi-dimensional data structures effectively. In Kotlin, nested loops provide powerful capabilities for handling complex scenarios while maintaining code readability. This comprehensive guide explores nested loops in Kotlin, their implementation, use cases, and best practices.
What are Nested Loops?
A nested loop occurs when one loop is placed inside another loop. The inner loop completes all its iterations for each single iteration of the outer loop. This creates a multiplicative effect on the total number of iterations, making nested loops both powerful and potentially resource-intensive.
Types of Nested Loops in Kotlin
1. Nested For Loops
The most common type of nested loop involves using Kotlin’s for loops together. Here’s a basic structure:
for (i in 1..3) {
for (j in 1..3) {
println("i: $i, j: $j")
}
}
2. Nested While Loops
While loops can also be nested, offering more flexibility in terms of iteration control:
var i = 1
while (i <= 3) {
var j = 1
while (j <= 3) {
println("i: $i, j: $j")
j++
}
i++
}
3. Mixed Nested Loops
Kotlin allows mixing different types of loops for maximum flexibility:
for (i in 1..3) {
var j = 1
while (j <= 3) {
println("i: $i, j: $j")
j++
}
}
Common Use Cases
1. Working with Multi-dimensional Arrays
Nested loops are essential for processing multi-dimensional arrays:
fun process2DArray() {
val matrix = arrayOf(
arrayOf(1, 2, 3),
arrayOf(4, 5, 6),
arrayOf(7, 8, 9)
)
for (row in matrix.indices) {
for (col in matrix[row].indices) {
println("Element at [$row][$col]: ${matrix[row][col]}")
}
}
}
2. Pattern Printing
Nested loops are frequently used to print various patterns:
fun printTriangle(height: Int) {
for (i in 1..height) {
for (j in 1..i) {
print("* ")
}
println()
}
}
3. Data Processing and Transformation
When processing complex data structures or performing data transformations:
data class Student(val name: String, val courses: List<String>)
fun processStudentData(students: List<Student>) {
for (student in students) {
println("Student: ${student.name}")
for (course in student.courses) {
println(" - Enrolled in: $course")
}
}
}
Performance Considerations and Optimization
1. Time Complexity
Nested loops multiply the number of iterations, affecting performance:
// O(n²) time complexity
fun quadraticTimeExample(n: Int) {
for (i in 1..n) {
for (j in 1..n) {
// Each operation here runs n * n times
println("Operation at i=$i, j=$j")
}
}
}
2. Memory Usage
Proper memory management is crucial when working with nested loops:
fun efficientProcessing(data: List<List<Int>>) {
// Use sequence for large datasets to minimize memory usage
data.asSequence().forEach { outerList ->
outerList.asSequence().forEach { element ->
processElement(element)
}
}
}
3. Loop Optimization Techniques
Several techniques can improve nested loop performance:
// Loop fusion - combining similar loops
fun optimizedProcessing(matrix: Array<Array<Int>>) {
for (i in matrix.indices) {
for (j in matrix[i].indices) {
// Process multiple operations in a single loop
matrix[i][j] = processValue(matrix[i][j])
validateValue(matrix[i][j])
transformValue(matrix[i][j])
}
}
}
Best Practices
1. Maintain Clear Loop Variables
Use meaningful names for loop variables to improve code readability:
fun processCustomerOrders(customers: List<Customer>) {
for (customer in customers) {
for (order in customer.orders) {
for (item in order.items) {
// Clear variable names make the code self-documenting
processOrderItem(customer, order, item)
}
}
}
}
2. Control Loop Depth
Limit the depth of nested loops to maintain code clarity:
// Consider refactoring deeply nested loops
fun processData(data: List<List<List<Int>>>) {
data.forEach { outerList ->
processOuterList(outerList)
}
}
private fun processOuterList(outerList: List<List<Int>>) {
outerList.forEach { innerList ->
processInnerList(innerList)
}
}
private fun processInnerList(innerList: List<Int>) {
innerList.forEach { element ->
processElement(element)
}
}
3. Use Loop Labels
Kotlin provides loop labels for better control in nested loops:
fun searchMatrix(matrix: Array<Array<Int>>, target: Int) {
outer@ for (i in matrix.indices) {
for (j in matrix[i].indices) {
if (matrix[i][j] == target) {
println("Found at position [$i][$j]")
break@outer // Breaks out of both loops
}
}
}
}
Common Pitfalls and Solutions
1. Infinite Loops
Ensure proper termination conditions:
// Potential infinite loop
fun riskyNestedLoop() {
var i = 0
var j = 0
while (i < 5) {
while (j < 5) {
println("$i, $j")
j++
}
i++
// j should be reset here
}
}
// Corrected version
fun safeNestedLoop() {
var i = 0
while (i < 5) {
var j = 0
while (j < 5) {
println("$i, $j")
j++
}
i++
}
}
2. Resource Management
Properly handle resources in nested loops:
fun processFiles(directories: List<File>) {
directories.forEach { dir ->
dir.listFiles()?.forEach { file ->
file.bufferedReader().use { reader ->
reader.lineSequence().forEach { line ->
processLine(line)
}
}
}
}
}
Conclusion
Nested loops in Kotlin are a powerful tool for handling complex iterations and data structures. While they can be resource-intensive, proper implementation and adherence to best practices can help you write efficient and maintainable code. Remember to consider performance implications, maintain code readability, and choose the appropriate loop structure for your specific use case.
Understanding when and how to use nested loops effectively is crucial for any Kotlin developer. By following the guidelines and examples provided in this guide, you can make better decisions about implementing nested loops in your projects while avoiding common pitfalls and performance issues.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.