Open Classes in Kotlin Programming Language
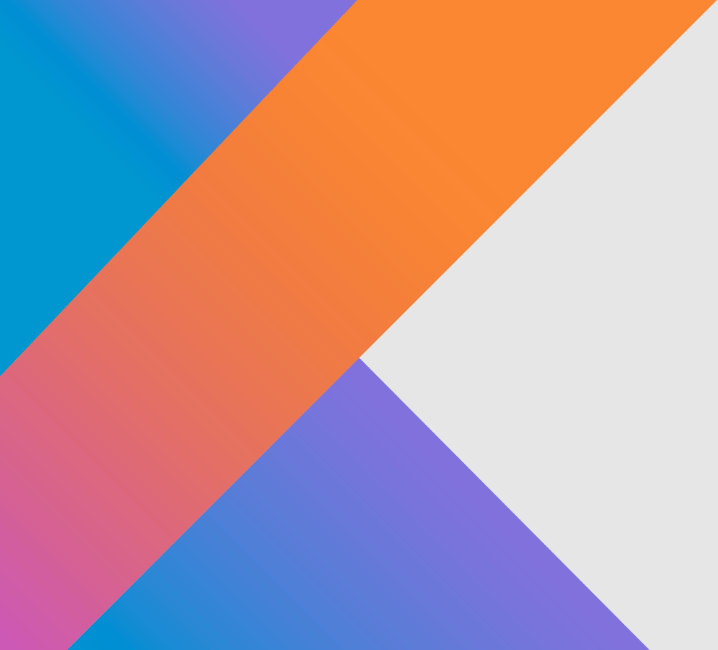
Categories:
4 minute read
Kotlin is a modern, concise, and powerful programming language that has gained immense popularity, especially for Android development. One of Kotlin’s defining features is its approach to object-oriented programming (OOP), particularly how it handles class inheritance through the open
keyword. In this article, we will explore open classes in Kotlin, why they are necessary, how they work, and how they compare to other OOP paradigms in different languages.
Understanding Open Classes in Kotlin
In Kotlin, classes are final by default. This means that unless explicitly stated otherwise, a class cannot be inherited. This is a major shift from Java, where all classes are open for inheritance unless marked as final
.
To allow a class to be inheritable in Kotlin, you must explicitly declare it as open
. This design decision enforces better software architecture by preventing unintended class extension and promoting composition over inheritance.
Syntax of Open Classes
To declare an open class in Kotlin, use the open
keyword:
open class Animal {
open fun makeSound() {
println("Some generic animal sound")
}
}
class Dog : Animal() {
override fun makeSound() {
println("Bark!")
}
}
fun main() {
val myDog = Dog()
myDog.makeSound() // Output: Bark!
}
Explanation
- The
Animal
class is marked asopen
, making it inheritable. - The
makeSound()
method is also markedopen
, allowing subclasses to override it. - The
Dog
class extendsAnimal
and provides its own implementation ofmakeSound()
.
Why Are Kotlin Classes Final by Default?
Kotlin’s decision to make classes final by default promotes better software design by preventing accidental inheritance. This is aligned with the principle of favoring composition over inheritance, which helps to avoid common issues such as deep inheritance hierarchies and unintended modifications.
Some benefits of this approach include:
- Encapsulation & Maintainability: Preventing unnecessary inheritance helps maintain encapsulation.
- Improved Performance: The compiler can optimize final classes better than open ones.
- Predictable Behavior: Code remains predictable and less prone to accidental modifications.
Open vs. Final vs. Abstract Classes
Kotlin provides three primary ways to define classes with different inheritance rules:
Modifier | Description |
---|---|
final (default) | Class cannot be inherited. |
open | Class can be inherited. |
abstract | Must be inherited; cannot be instantiated directly. |
Let’s compare these with an example:
// Final class (default)
class Vehicle {
fun drive() {
println("Driving...")
}
}
// Open class
open class Car {
open fun honk() {
println("Beep beep!")
}
}
// Abstract class
abstract class Plane {
abstract fun fly()
}
class Boeing747 : Plane() {
override fun fly() {
println("Flying high!")
}
}
Overriding Methods in Open Classes
In addition to marking a class as open
, you must also explicitly mark methods or properties as open
to allow them to be overridden in subclasses.
open class Person {
open val name: String = "Unknown"
open fun introduce() {
println("Hi, my name is $name")
}
}
class Student : Person() {
override val name: String = "Alice"
override fun introduce() {
println("I am a student, my name is $name")
}
}
fun main() {
val student = Student()
student.introduce() // Output: I am a student, my name is Alice
}
Open Properties and Their Behavior
When a property is marked as open
, it can be overridden in derived classes. However, if a property is declared as val
(read-only), it can only be overridden by another val
, while a var
can be overridden by either a val
or var
.
open class Parent {
open val info: String = "Parent Info"
}
class Child : Parent() {
override val info: String = "Child Info"
}
fun main() {
val obj = Child()
println(obj.info) // Output: Child Info
}
Sealed Classes: A Restrictive Alternative
If you want to limit inheritance to a specific set of subclasses, you can use sealed classes instead of open
classes. A sealed class is implicitly open
but only allows inheritance within the same file.
sealed class Result {
class Success(val data: String) : Result()
class Error(val message: String) : Result()
}
fun handleResult(result: Result) {
when (result) {
is Result.Success -> println("Success: ${result.data}")
is Result.Error -> println("Error: ${result.message}")
}
}
Best Practices for Using Open Classes
- Use
open
only when necessary – Don’t mark every class asopen
. Instead, use composition or interfaces where applicable. - Prefer sealed classes for limited inheritance – If you only want a fixed set of subclasses, consider using
sealed
instead ofopen
. - Override methods wisely – Ensure overridden methods preserve the integrity of the base class behavior.
- Encapsulate implementation details – If certain methods should not be modified, avoid marking them as
open
.
Conclusion
Open classes in Kotlin provide a controlled way of enabling inheritance, ensuring better maintainability and code safety. By making classes final by default, Kotlin encourages developers to think carefully before allowing inheritance, leading to cleaner and more robust codebases. By understanding open
classes, sealed
classes, and overriding behaviors, you can design efficient object-oriented programs in Kotlin that are both flexible and maintainable.
Whether you’re building Android applications or backend services with Kotlin, using open
classes effectively will enhance your ability to create scalable and well-structured software solutions.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.