Pseudocode: A Blueprint for Algorithms
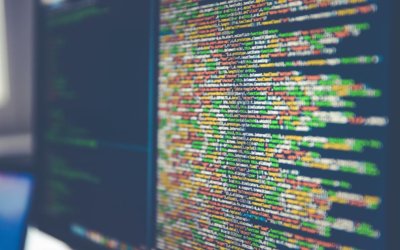
Categories:
3 minute read
Introduction
Pseudocode is a simplified, informal language used to describe algorithms and programming logic. It’s a valuable tool for planning and communicating the steps involved in a problem-solving process. Unlike actual programming languages, pseudocode doesn’t adhere to strict syntax rules, making it easier to understand and write.
Key Characteristics of Pseudocode
Clarity and Conciseness: Pseudocode should be easy to read and understand, even for those unfamiliar with programming. It avoids unnecessary details and focuses on the core logic of the algorithm.
Modularity: Pseudocode often breaks down algorithms into smaller, more manageable steps or modules. This makes it easier to analyze, debug, and modify the code.
Abstraction: Pseudocode abstracts away from the specific syntax of a programming language, allowing you to focus on the algorithmic concepts.
Independence: Pseudocode is not tied to a particular programming language, making it a versatile tool for describing algorithms that can be implemented in various languages. Basic Pseudocode Constructs
Sequential Execution: Instructions are executed one after another, in the order they appear.
Decision Making: The
if-else
statement is used to make decisions based on conditions.Iteration: Loops like
for
,while
, anddo-while
are used to repeat a block of code multiple times.Procedures and Functions: Subroutines that can be called from other parts of the code.
Comments: Used to explain the purpose of specific code sections. Example of Pseudocode
Here’s a simple example of pseudocode for a bubble sort algorithm:
function bubbleSort(array)
for i = 0 to array.length - 1
for j = 0 to array.length - i - 1
if array[j] > array[j+1]
swap array[j] and array[j+1]
Benefits of Using Pseudocode
Algorithm Planning: Pseudocode helps in planning and designing algorithms by providing a clear outline of the steps involved.
Communication: It serves as a common language for communicating algorithmic ideas among programmers and non-programmers.
Debugging: Pseudocode can be used to identify and correct errors in algorithms before implementing them in a programming language.
Code Generation: Once the pseudocode is finalized, it can be translated into a specific programming language.
Learning Aid: Pseudocode is a valuable tool for learning programming concepts and understanding how algorithms work. Best Practices for Writing Pseudocode
Use Consistent Indentation: Indentation helps to visually represent the structure of the code.
Choose Descriptive Variable Names: Use meaningful names that reflect the purpose of variables.
Add Comments: Explain the purpose of complex sections or algorithms.
Break Down Complex Problems: Divide large problems into smaller, more manageable subproblems.
Test Your Pseudocode: Run through the pseudocode with sample inputs to ensure it produces the correct output. Common Pseudocode Constructs and Their Equivalents in Programming Languages
Pseudocode Construct | C++ Equivalent | Python Equivalent | Java Equivalent |
---|---|---|---|
`if-else` | `if-else` | `if-else` | `if-else` |
`for` loop | `for` loop | `for` loop | `for` loop |
`while` loop | `while` loop | `while` loop | `while` loop |
`do-while` loop | `do-while` loop | `while True:` (with `break` statement) | `do-while` loop |
`function` | `function` | `def` | `method` |
`procedure` | `void` function | `def` | `void` method |
Conclusion
Pseudocode is a valuable tool for understanding, designing, and communicating algorithms. By following the guidelines outlined in this blog post, you can effectively use pseudocode to enhance your problem-solving skills and improve your programming abilities.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.