Securing APIs: Best Practices for Developers and Security Teams
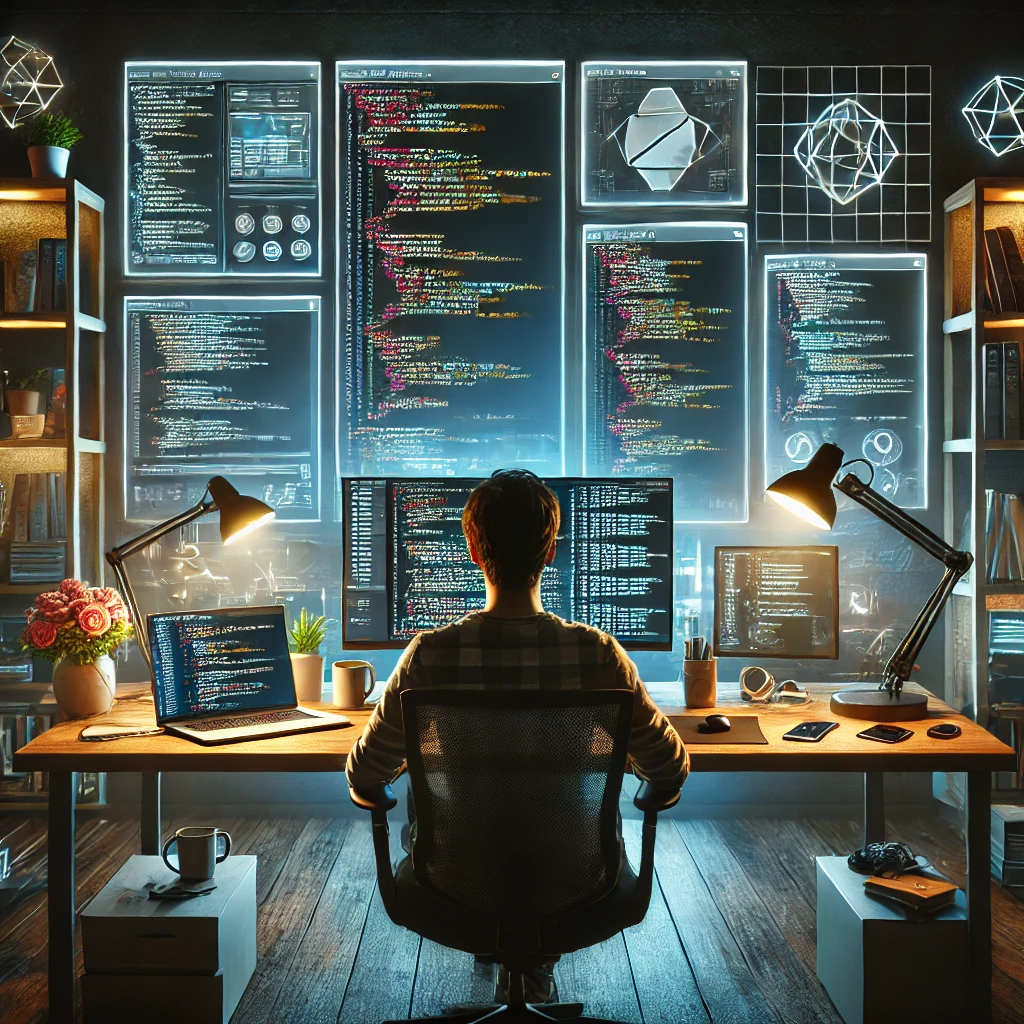
Categories:
9 minute read
In today’s interconnected digital landscape, Application Programming Interfaces (APIs) have become the backbone of modern software development. APIs allow different applications to communicate, share data, and leverage each other’s functionalities. They power everything from mobile apps to cloud services, making it possible for developers to build complex applications by integrating third-party services. However, as the adoption of APIs increases, so do the security risks associated with them.
APIs are frequent targets for cyberattacks, and if left unprotected, they can expose sensitive data, enable unauthorized access, and create vulnerabilities in applications. For this reason, securing APIs is essential not only for developers but also for security teams tasked with protecting applications and data from cyber threats.
In this blog, we will explore the best practices for securing APIs, covering both technical measures and organizational strategies that can help developers and security teams collaborate effectively to build and maintain secure systems.
Why API Security is Critical
The increasing dependence on APIs to connect various services and applications means they are an attractive target for attackers. According to recent security reports, API-based attacks are on the rise, with many organizations lacking the necessary defenses to protect their systems. Some of the main reasons why API security is so critical include:
APIs Expose Business Logic: Unlike traditional web applications that primarily expose user interfaces, APIs expose business logic directly. If an attacker gains access to an API, they can manipulate the core functions of an application.
Sensitive Data Exposure: APIs often handle sensitive data such as personal information, payment details, and authentication tokens. If APIs are not properly secured, attackers can intercept and steal this data.
Increasing API Usage: As more companies adopt API-driven architectures, the attack surface grows, making APIs a primary entry point for cybercriminals.
API Misconfigurations: API vulnerabilities often result from misconfigurations, such as overly permissive access controls, weak authentication, or lack of encryption. Given the risks associated with APIs, developers and security teams must work together to implement best practices for securing API endpoints and ensuring robust protection against potential threats.
Best Practices for Securing APIs
- Use Strong Authentication and Authorization
One of the most critical steps in securing APIs is to ensure that only authorized users and services can access them. This requires robust authentication and authorization mechanisms.
OAuth 2.0: OAuth 2.0 is a widely adopted authorization framework that allows third-party applications to access a user’s resources without exposing credentials. OAuth 2.0 is commonly used for securing APIs in both public and internal systems. It uses access tokens to represent user permissions and allows users to control what data is shared with external applications.
OpenID Connect (OIDC): OIDC is built on top of OAuth 2.0 and adds an identity layer to enable secure user authentication. It provides a standardized way of verifying the identity of users accessing APIs, ensuring that the API is only accessible to authenticated users.
JWT (JSON Web Tokens): JWTs are commonly used for securing APIs by encoding user information in a token. These tokens are signed to ensure their authenticity and are passed between the client and the API to authenticate requests. However, it is essential to configure JWTs correctly, including setting appropriate expiration times and signing tokens with secure algorithms.
API Keys: API keys are another form of authentication commonly used to control access to APIs. However, API keys alone are often insufficient for securing sensitive APIs because they don’t verify the identity of the user. API keys should always be combined with other forms of authentication, such as OAuth or JWT.
- Use HTTPS for Secure Communication
APIs often transmit sensitive data, and securing this data in transit is crucial. Always use HTTPS to encrypt data between the client and the server, preventing attackers from intercepting sensitive information such as passwords or access tokens.
TLS (Transport Layer Security): TLS is the cryptographic protocol that secures HTTPS. Ensure that your APIs are configured to use the latest version of TLS (preferably TLS 1.3) and disable older, insecure versions such as TLS 1.0 and 1.1.
Strict Transport Security: Implement HTTP Strict Transport Security (HSTS) to ensure that browsers and clients only make secure HTTPS connections to your API endpoints. This helps prevent downgrade attacks, where an attacker forces a connection to use an insecure version of TLS.
- Rate Limiting and Throttling
Rate limiting is a technique used to control the number of requests an API can handle over a given period. By implementing rate limiting, you can prevent denial-of-service (DoS) attacks and abuse of your API by malicious users or even well-intentioned users who make too many requests in a short time.
- Throttling: Throttling works alongside rate limiting by controlling the rate at which requests are processed. This ensures that the API can handle large bursts of traffic without degrading performance. Rate limiting and throttling can be applied at various levels, such as per user, per IP address, or per API key, ensuring that the API is not overwhelmed by too many requests.
- Validate and Sanitize Input
One of the most common vulnerabilities in APIs is improper input validation, which can lead to attacks such as SQL injection, command injection, and cross-site scripting (XSS). Attackers often exploit weak input validation to manipulate API requests and gain unauthorized access to resources.
To mitigate these risks:
Validate Input: Always validate incoming data to ensure that it conforms to the expected format, type, and length. For example, if an API expects a user ID to be an integer, ensure that only numeric values are accepted.
Sanitize Data: Remove or neutralize any potentially harmful content from input data, such as special characters or executable code. This prevents attackers from injecting malicious payloads into API requests.
Schema Validation: Use a schema validation tool, such as JSON Schema for RESTful APIs, to enforce the expected structure of the input data. This ensures that all incoming requests adhere to the API’s expected schema and format.
- Implement Robust Error Handling
When APIs return detailed error messages, they can inadvertently expose sensitive information, such as database queries, stack traces, or internal logic, that attackers can use to exploit vulnerabilities. To prevent this:
Generic Error Messages: Ensure that APIs return generic error messages that do not reveal internal details about the system. For example, instead of returning “SQL error: column not found,” use a more generic message such as “Server error.”
Status Codes: Use appropriate HTTP status codes to indicate the nature of the error without exposing sensitive information. For example, return a 401 status code for unauthorized access or a 400 status code for invalid input.
- Use API Gateways for Centralized Security
An API gateway acts as a single entry point for all API traffic and provides several security-related features that help enforce best practices across all APIs. API gateways handle tasks such as:
Authentication and Authorization: Centralized authentication, authorization, and access control for all API endpoints.
Traffic Monitoring: Monitoring incoming requests, identifying anomalies, and applying rate limiting or throttling as needed.
Request/Response Transformation: Filtering and transforming requests or responses to ensure compliance with security policies. By using an API gateway, you can manage security at scale, enforce security policies consistently, and simplify the deployment and maintenance of secure APIs.
- Monitor API Traffic and Logging
Proactive monitoring is a vital aspect of API security. By monitoring and logging API traffic, you can detect potential security incidents, such as unusual traffic patterns, unauthorized access attempts, or abuse of the API.
API Monitoring: Set up real-time monitoring for your APIs using tools like Prometheus, Grafana, or your cloud provider’s monitoring service (e.g., AWS CloudWatch or Azure Monitor). This will help you track key metrics, such as request rates, error rates, and latency.
Centralized Logging: Ensure that all API activity is logged in a centralized system for easy analysis. Log data should include information such as timestamps, IP addresses, user activity, and error messages. Centralized logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk can help you detect anomalies and investigate potential breaches.
Audit Logs: Use audit logs to keep track of user access and system changes. This can be useful for identifying potential threats and investigating any security incidents.
- Encrypt Sensitive Data
API requests often handle sensitive data, such as user credentials, financial information, or personal data. To protect this data from unauthorized access, it is essential to implement encryption at multiple layers:
Data at Rest: Ensure that sensitive data stored in databases or file systems is encrypted using strong encryption algorithms, such as AES-256.
Data in Transit: As mentioned earlier, always use HTTPS to encrypt data transmitted between the API client and server.
Sensitive Field Masking: When handling particularly sensitive information, such as credit card numbers or Social Security numbers, consider masking or redacting these fields before storing or transmitting them. This ensures that sensitive data is protected even if an attacker gains access to the API traffic or logs.
- Regularly Test and Audit APIs
Regular security testing and auditing are crucial for maintaining secure APIs. Some key practices include:
Penetration Testing: Hire security professionals to conduct penetration tests on your APIs. These tests simulate real-world attacks and help identify vulnerabilities that might not be obvious during development.
Vulnerability Scanning: Use automated tools to scan your APIs for known vulnerabilities, such as OWASP’s Top 10 API Security Risks, which includes issues like broken authentication, excessive data exposure, and lack of resource rate limiting.
Code Reviews: Perform regular code reviews, focusing on security aspects of API development. Peer reviews can help catch potential security flaws early in the development process.
- Keep Software and Dependencies Up to Date
Finally, keeping your software, libraries, and dependencies up to date is essential for maintaining security. Many API vulnerabilities arise from outdated libraries or software with known security flaws.
Dependency Management: Use tools like npm audit (for JavaScript) or Snyk to monitor and manage third-party libraries and dependencies. Regularly update these dependencies to patch any security vulnerabilities.
Patch Management: Ensure that security patches and updates are applied promptly to all systems, including API gateways, servers, and infrastructure.
Conclusion
Securing APIs is a critical task for developers and security teams as APIs continue to play a pivotal role in modern application development. By following best practices, such as implementing strong authentication, using HTTPS, validating inputs, and regularly monitoring API traffic, organizations can protect their APIs from unauthorized access, data breaches, and other security threats.
By fostering collaboration between development and security teams, organizations can integrate security into the API development lifecycle, ensuring that APIs remain secure as they scale. With the right tools, policies, and awareness, developers and security teams can work together to build robust, secure, and resilient APIs that stand the test of time in today’s rapidly evolving cyber threat landscape.