Understanding Kotlin's Scope Functions apply and also
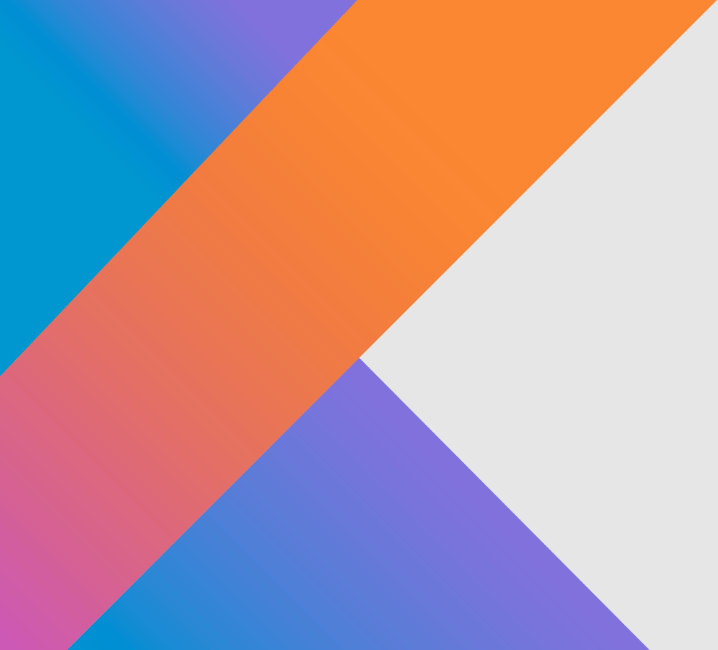
Categories:
4 minute read
Continuing our exploration of Kotlin’s scope functions, we’ll dive deep into apply
and also
- two powerful functions that complement the previously discussed scope functions. These functions provide unique ways to handle object configuration and side effects in your Kotlin code.
The ‘apply’ Scope Function
The apply
function is particularly useful for object configuration and initialization. It operates on a context object and returns the object itself after applying the specified operations.
Basic Syntax
val result = object.apply {
// 'this' refers to the object
// returns the object itself
}
Key Characteristics
- Context Object: Available as ’this’
- Return Value: Context object (receiver object)
- Use Case: Object configuration and initialization
Practical Examples
// Object configuration
val textView = TextView(context).apply {
text = "Hello, World!"
textSize = 16f
setTextColor(Color.BLACK)
setPadding(16, 16, 16, 16)
}
// Complex object initialization
data class Person(
var name: String = "",
var age: Int = 0,
var address: String = ""
)
val person = Person().apply {
name = "Alice"
age = 25
address = "456 Oak Street"
}
// Builder pattern alternative
val urlConnection = URL("https://example.com").openConnection().apply {
connectTimeout = 3000
readTimeout = 5000
doInput = true
setRequestProperty("Content-Type", "application/json")
}
Advanced Usage Patterns
Chaining Configuration
class Configuration {
val settings = mutableMapOf<String, Any>()
fun configure() = apply {
settings["timeout"] = 5000
settings["retries"] = 3
settings["baseUrl"] = "https://api.example.com"
}
fun setEnvironment(env: String) = apply {
settings["environment"] = env
}
}
val config = Configuration()
.configure()
.setEnvironment("production")
Working with Collections
val mutableList = mutableListOf<String>().apply {
add("First")
add("Second")
addAll(listOf("Third", "Fourth"))
shuffle()
}
The ‘also’ Scope Function
The also
function is perfect for performing additional operations or side effects in a chain of operations. It’s similar to apply
but provides the context object as a lambda parameter.
Basic Syntax
val result = object.also {
// 'it' refers to the object
// returns the object itself
}
Key Characteristics
- Context Object: Available as ‘it’ (can be renamed)
- Return Value: Context object (receiver object)
- Use Case: Additional actions, logging, debugging
Practical Examples
// Logging during chain operations
data class User(val id: Int, var name: String)
val user = User(1, "John")
.also { println("Created user: $it") }
.also { log.debug("User details: $it") }
// Validation with side effects
fun processUser(user: User) = user.also {
require(it.name.isNotBlank()) { "User name cannot be blank" }
require(it.id > 0) { "Invalid user ID" }
}
// Debugging in call chains
val numbers = mutableListOf<Int>()
.also { println("Initial list: empty") }
.apply { add(1) }
.also { println("After adding 1: $it") }
.apply { add(2) }
.also { println("After adding 2: $it") }
Advanced Applications
Intermediate Processing
class DataProcessor {
fun process(data: List<String>) = data
.map { it.uppercase() }
.also { intermediateList ->
println("After uppercase: $intermediateList")
saveToLog(intermediateList)
}
.filter { it.length > 3 }
.also { filteredList ->
println("After filtering: $filteredList")
updateMetrics(filteredList.size)
}
}
Object Registration
class ComponentRegistry {
private val components = mutableListOf<Component>()
fun register(component: Component) = component.also {
components.add(it)
notifyListeners(ComponentEvent.REGISTERED, it)
}
}
Comparing apply and also
Understanding when to use apply
versus also
is crucial for writing clean and maintainable code.
Key Differences
Context Object Access
apply
: Uses ’this’ (implicit receiver)also
: Uses ‘it’ (explicit parameter)
Typical Use Cases
apply
: Object configuration and initializationalso
: Side effects and logging
Code Style
apply
: More concise when calling methods on the objectalso
: More explicit and clear for external operations
Decision Guidelines
Choose apply
when:
- Configuring object properties
- Initializing objects
- Working with builder-style APIs
- Calling multiple methods on the same object
Choose also
when:
- Adding logging or debugging steps
- Performing validation
- Adding side effects to a chain of operations
- Need to reference the object explicitly
Best Practices
Clear Intent
- Use
apply
for configuration - Use
also
for side effects - Don’t mix concerns within the same block
- Use
Scope Size
- Keep blocks small and focused
- Extract complex logic into separate functions
Readability
- Avoid nesting scope functions
- Use meaningful names when referencing objects
- Add comments for complex chains
Chain Organization
- Place
also
blocks strategically for logging - Group related operations in
apply
blocks
- Place
Common Pitfalls to Avoid
Overuse
- Don’t use scope functions for simple operations
- Avoid creating unnecessary blocks
Mixing Concerns
- Keep configuration and side effects separate
- Don’t combine different responsibilities
Complex Nesting
- Avoid deep nesting of scope functions
- Break down complex operations
Conclusion
The apply
and also
scope functions are powerful tools in Kotlin that serve distinct purposes. apply
excels at object configuration and initialization, while also
is perfect for adding side effects and debugging steps to your code. Understanding their differences and appropriate use cases will help you write more expressive and maintainable Kotlin code.
Remember that while these functions can make your code more concise and readable, they should be used judiciously. The key is to maintain clarity and purpose in your code while leveraging these powerful features effectively.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.