Understanding Kotlin Scope Functions: `let`, `run`, and `with`
let
, run
, and with
- provide a clean and concise way to execute code blocks within the context of an object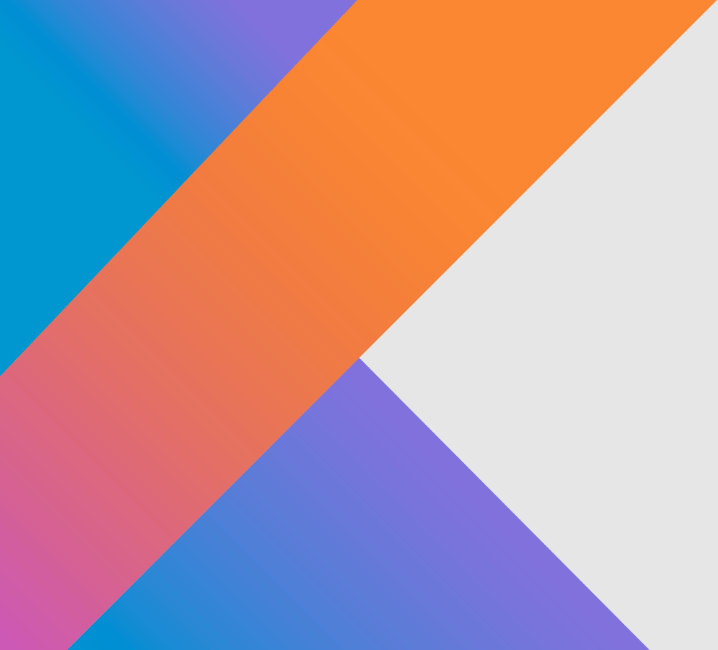
Categories:
4 minute read
Kotlin’s scope functions are powerful features that provide a clean and concise way to execute code blocks within the context of an object. In this comprehensive guide, we’ll explore three essential scope functions - let
, run
, and with
- understanding their purposes, differences, and best practices for implementation.
Introduction to Scope Functions
Scope functions are unique to Kotlin and create a temporary scope where you can access an object without explicitly naming it. They serve different purposes and can significantly improve code readability and maintainability when used appropriately.
The ’let’ Scope Function
The let
function is perhaps the most commonly used scope function in Kotlin. It takes the object it is invoked upon as an argument and returns the result of the lambda expression.
Basic Syntax
val result = object.let {
// 'it' refers to the object
// last expression is the return value
}
Key Characteristics
- Context Object: Available as ‘it’ (can be renamed)
- Return Value: Lambda result
- Use Case: Executing code block with non-null values and introducing local variables
Practical Examples
// Null check and operation
nullable?.let {
println("Value is not null: $it")
}
// Chain operations
val numbers = listOf("one", "two", "three")
val modifiedNumbers = numbers.map { it.uppercase() }
.let { modifiedList ->
modifiedList.filter { it.length > 3 }
}
// Transform and assign
val length = str?.let {
println("Processing string: $it")
it.length
} ?: 0
The ‘run’ Scope Function
The run
function is similar to let
but handles the context object differently. It’s particularly useful when you need to initialize an object and compute a result.
Basic Syntax
val result = object.run {
// 'this' refers to the object
// last expression is the return value
}
Key Characteristics
- Context Object: Available as ’this’
- Return Value: Lambda result
- Use Case: Object initialization and computing results
Practical Examples
// Initialize and configure an object
val service = NetworkService().run {
port = 8080
connect()
this // return the configured object
}
// Complex calculations with context
val result = account.run {
if (balance < 0) {
throw IllegalStateException("Balance cannot be negative")
}
balance * interestRate + calculateBonus()
}
// Multiple operations on an object
val textConfig = TextProperties().run {
fontSize = 14
fontFamily = "Arial"
opacity = 0.95f
toString() // return string representation
}
The ‘with’ Scope Function
The with
function is slightly different as it takes the context object as an argument rather than being called on the object itself. It’s ideal for grouping multiple operations on an object.
Basic Syntax
val result = with(object) {
// 'this' refers to the object
// last expression is the return value
}
Key Characteristics
- Context Object: Available as ’this’
- Return Value: Lambda result
- Use Case: Grouping multiple operations on an object
Practical Examples
// Configure multiple properties
with(person) {
name = "John"
age = 30
address = "123 Main St"
}
// Complex object manipulation
val dimensions = with(rectangle) {
println("Processing rectangle")
val area = width * height
val perimeter = 2 * (width + height)
"Area: $area, Perimeter: $perimeter"
}
// Working with builders
val htmlString = with(StringBuilder()) {
append("<html>")
append("<body>")
append("<h1>Hello, World!</h1>")
append("</body>")
append("</html>")
toString()
}
Choosing Between Scope Functions
When deciding which scope function to use, consider these factors:
Context Object Reference
- Use
let
when you prefer using ‘it’ or want to rename the context object - Use
run
orwith
when you prefer using ’this’ and calling object methods directly
- Use
Return Value Needs
- All three return the lambda result
- Choose based on whether you need to transform the object or perform operations
Null Safety
let
is particularly useful for null safety checks with the safe call operator (?.)run
andwith
are better for non-null objects
Best Practices
Keep Lambda Bodies Concise
- Avoid lengthy blocks of code within scope functions
- Break down complex operations into smaller functions
Choose Meaningful Names
- When using
let
, rename ‘it’ if the lambda is complex or nested - Use descriptive variable names for clarity
- When using
Consider Readability
- Don’t nest scope functions deeply
- Use regular functions if the scope function makes code harder to understand
Consistent Usage
- Establish team conventions for scope function usage
- Document unusual or complex applications
Conclusion
Kotlin’s scope functions - let
, run
, and with
- are powerful tools that can make your code more concise and expressive. Each has its specific use cases and advantages. Understanding their differences and applying them appropriately will help you write more maintainable and readable Kotlin code.
Remember that while these functions can greatly improve code clarity, they should be used judiciously. The goal is to enhance readability and maintainability, not to make the code more complex or harder to understand.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.