Understanding Lambda Syntax in Kotlin Programming Language
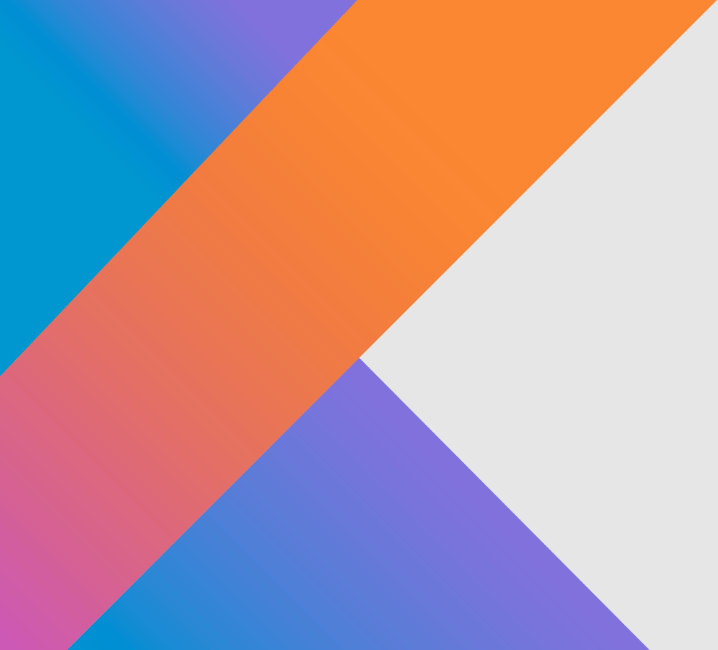
Categories:
4 minute read
Kotlin, developed by JetBrains, is a modern programming language that runs on the Java Virtual Machine (JVM) and is fully interoperable with Java. One of the most powerful features of Kotlin is its support for functional programming, including lambda expressions. Lambda expressions, or simply lambdas, allow for concise and expressive code, making development faster and more readable. In this blog post, we will delve deep into the lambda syntax in Kotlin, exploring its structure, usage, and best practices.
What is a Lambda Expression?
A lambda expression is an anonymous function that can be treated as a value. It enables functional programming by allowing functions to be passed as arguments, returned from other functions, or stored in variables. In essence, a lambda provides a succinct way to define and use functions without explicitly declaring them.
Basic Syntax of a Lambda
In Kotlin, a lambda expression is defined using the following syntax:
val lambdaName: (InputType) -> ReturnType = { argument: InputType -> expression }
Here’s a breakdown of the components:
val lambdaName
: Declares a lambda as a variable.(InputType) -> ReturnType
: Specifies the function signature, defining input and output types.{ argument: InputType -> expression }
: The actual lambda function.
Example of a Simple Lambda
val square: (Int) -> Int = { number: Int -> number * number }
println(square(4)) // Output: 16
In this example, square
is a lambda that takes an integer and returns its square.
Implicit Type Inference in Lambdas
Kotlin’s type inference mechanism can infer the type of arguments and return values, allowing us to write more concise code. For example:
val square = { number: Int -> number * number }
The compiler automatically infers that square
is of type (Int) -> Int
, so we don’t need to explicitly specify it.
Multi-Parameter Lambdas
Lambdas can accept multiple parameters, separated by commas.
val sum: (Int, Int) -> Int = { a, b -> a + b }
println(sum(3, 5)) // Output: 8
The it
Keyword in Kotlin Lambdas
If a lambda has only one parameter, Kotlin allows us to refer to it implicitly using the it
keyword. This makes the lambda even more concise.
val double = { it * 2 }
println(double(6)) // Output: 12
Here, it
represents the single argument passed to the lambda.
Higher-Order Functions and Lambdas
A higher-order function is a function that takes another function as a parameter or returns a function. Kotlin’s standard library provides several higher-order functions, such as map
, filter
, and forEach
, that work with lambdas.
Example of a Higher-Order Function with a Lambda
fun operateOnNumbers(a: Int, b: Int, operation: (Int, Int) -> Int): Int {
return operation(a, b)
}
val result = operateOnNumbers(10, 5) { x, y -> x + y }
println(result) // Output: 15
Here, operateOnNumbers
takes two integers and a function (lambda) that specifies how to operate on them.
Lambda with Collections
Kotlin’s collections framework benefits greatly from lambdas.
Using map
with Lambda
The map
function applies a transformation to each element in a list.
val numbers = listOf(1, 2, 3, 4, 5)
val squaredNumbers = numbers.map { it * it }
println(squaredNumbers) // Output: [1, 4, 9, 16, 25]
Using filter
with Lambda
The filter
function selects elements based on a given predicate.
val evenNumbers = numbers.filter { it % 2 == 0 }
println(evenNumbers) // Output: [2, 4]
Using forEach
with Lambda
The forEach
function applies a lambda to each element in a collection.
numbers.forEach { println(it * 2) }
Returning Values from Lambdas
A lambda’s last expression is implicitly returned.
val multiply: (Int, Int) -> Int = { a, b -> a * b }
println(multiply(3, 4)) // Output: 12
If a return statement is explicitly needed within a lambda, Kotlin requires the return@
label.
val numbers = listOf(1, 2, 3, 4, 5)
numbers.forEach {
if (it == 3) return@forEach
println(it)
}
Here, return@forEach
ensures that only the lambda exits, rather than the entire function.
Lambda with Receiver (Function Literals with Receiver)
A lambda with receiver allows calling functions on a context object inside the lambda without explicitly referencing it.
val stringBuilderAction: StringBuilder.() -> Unit = {
append("Hello, ")
append("World!")
}
val result = StringBuilder().apply(stringBuilderAction).toString()
println(result) // Output: Hello, World!
This is commonly used in Kotlin’s DSLs (Domain Specific Languages).
Best Practices for Using Lambdas in Kotlin
- Use
it
for single-parameter lambdas – This makes the code concise. - Leverage type inference – Avoid redundant type declarations unless necessary.
- Break long lambda expressions into functions – Enhances readability and maintainability.
- Use function references (
::
) when possible – Improves clarity by reusing named functions.
fun square(num: Int) = num * num
val numbers = listOf(1, 2, 3, 4)
val squaredNumbers = numbers.map(::square)
println(squaredNumbers) // Output: [1, 4, 9, 16]
Conclusion
Lambdas are a key feature of Kotlin that enable concise and expressive functional programming. By understanding their syntax and best practices, developers can write more efficient and readable code. Whether used with collections, higher-order functions, or DSLs, mastering lambda expressions can significantly enhance Kotlin programming efficiency.
Happy coding!
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.