Understanding `map`, `filter`, and `reduce` in Kotlin
map
, filter
, and reduce
in Kotlin, their syntax, use cases, and practical examples.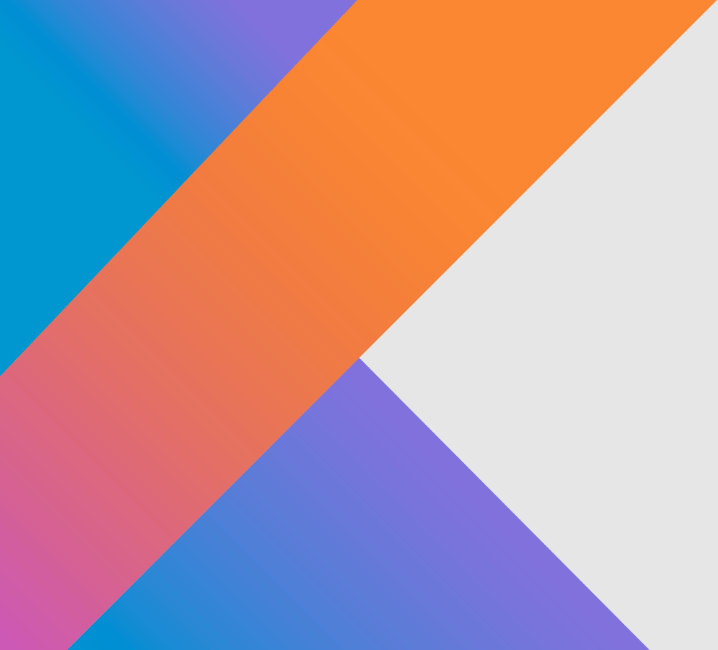
Categories:
4 minute read
Functional programming has gained immense popularity due to its concise, expressive, and efficient coding techniques. Kotlin, a modern programming language, embraces functional programming principles and provides a variety of higher-order functions to simplify common operations on collections. Among these functions, map
, filter
, and reduce
stand out as essential tools for transforming and processing data.
In this blog post, we will explore map
, filter
, and reduce
in Kotlin, understand their syntax and use cases, and see practical examples to demonstrate their power and efficiency.
1. The map
Function in Kotlin
The map
function is used to transform elements in a collection by applying a given function to each element. It returns a new collection containing the transformed elements.
Syntax
fun <T, R> Iterable<T>.map(transform: (T) -> R): List<R>
Example
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
val squaredNumbers = numbers.map { it * it }
println(squaredNumbers) // Output: [1, 4, 9, 16, 25]
}
In the above example, each element in the list is squared using map
, and a new list with transformed elements is created.
Use Cases
- Transforming a list of objects into another form (e.g., converting a list of integers to a list of strings).
- Extracting specific properties from a collection of objects.
- Performing mathematical operations on elements.
2. The filter
Function in Kotlin
The filter
function is used to select elements from a collection that satisfy a given condition. It returns a new collection containing only the elements that meet the specified predicate.
Syntax
fun <T> Iterable<T>.filter(predicate: (T) -> Boolean): List<T>
Example
fun main() {
val numbers = listOf(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
val evenNumbers = numbers.filter { it % 2 == 0 }
println(evenNumbers) // Output: [2, 4, 6, 8, 10]
}
In this example, filter
is used to extract only the even numbers from the list.
Use Cases
- Filtering out unwanted elements from a list.
- Selecting specific records from a collection of objects based on conditions.
- Removing null or empty values from a list.
3. The reduce
Function in Kotlin
The reduce
function is used to aggregate elements in a collection into a single value by applying a binary operation successively from left to right.
Syntax
fun <T> Iterable<T>.reduce(operation: (acc: T, T) -> T): T
Example
fun main() {
val numbers = listOf(1, 2, 3, 4, 5)
val sum = numbers.reduce { acc, num -> acc + num }
println(sum) // Output: 15
}
In this example, reduce
is used to compute the sum of all elements in the list.
Use Cases
- Accumulating values (sum, product, etc.).
- Concatenating strings or combining data.
- Calculating cumulative results from a dataset.
Combining map
, filter
, and reduce
Kotlin allows chaining of these functions to perform complex operations in a single statement.
Example
fun main() {
val numbers = listOf(1, 2, 3, 4, 5, 6, 7, 8, 9, 10)
val squaredSum = numbers.filter { it % 2 == 0 }
.map { it * it }
.reduce { acc, num -> acc + num }
println(squaredSum) // Output: 220 (sum of squares of even numbers)
}
Here, we first filter even numbers, then square them using map
, and finally sum them using reduce
.
Performance Considerations
While map
, filter
, and reduce
are powerful, they create intermediate collections that may impact performance, especially with large datasets. To optimize performance, Kotlin provides sequence processing.
Using Sequences
fun main() {
val numbers = (1..1000000).toList()
val result = numbers.asSequence()
.filter { it % 2 == 0 }
.map { it * it }
.reduce { acc, num -> acc + num }
println(result)
}
By converting the list to a sequence using asSequence()
, we avoid creating multiple intermediate collections, leading to improved performance.
Conclusion
The map
, filter
, and reduce
functions in Kotlin are essential tools for functional programming, enabling concise and efficient data transformations. Understanding these functions allows developers to write cleaner and more expressive code while working with collections.
Key Takeaways
map
transforms each element in a collection.filter
selects elements based on a condition.reduce
aggregates elements into a single value.- Chaining these functions allows powerful and concise data processing.
- Using sequences can improve performance for large datasets.
By mastering these functions, you can unlock the full potential of Kotlin’s functional programming capabilities and write more efficient, elegant, and readable code.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.