Understanding Tail Recursion in Kotlin
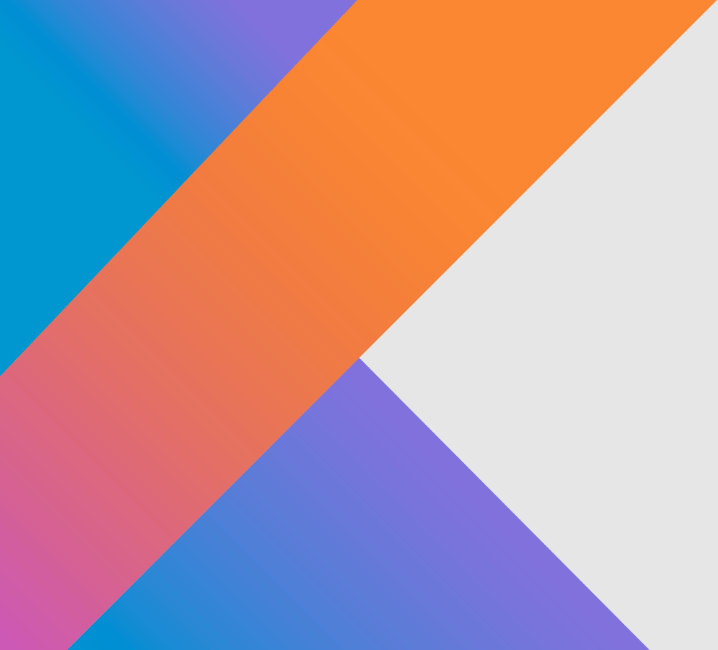
Categories:
5 minute read
Tail recursion is an important optimization technique in functional programming that Kotlin supports through its tailrec
modifier. This comprehensive guide explores tail recursion, its benefits, implementation, and best practices in Kotlin.
What is Tail Recursion?
Tail recursion is a special case of recursion where the recursive call is the last operation in a function. When a function is tail-recursive, the compiler can optimize it to use constant stack space, effectively converting the recursion into a loop.
Basic Syntax
tailrec fun factorial(n: Long, accumulator: Long = 1): Long {
return when (n) {
0L, 1L -> accumulator
else -> factorial(n - 1, n * accumulator)
}
}
Understanding the Difference
Regular Recursion vs Tail Recursion
// Regular recursion - Not tail-recursive
fun factorial1(n: Long): Long {
return if (n <= 1) 1
else n * factorial1(n - 1) // Must wait for recursive call to complete
}
// Tail recursion - Tail-recursive
tailrec fun factorial2(n: Long, accumulator: Long = 1): Long {
return when (n) {
0L, 1L -> accumulator
else -> factorial2(n - 1, n * accumulator) // Last operation is the recursive call
}
}
Benefits of Tail Recursion
1. Stack Safety
Prevents stack overflow for large recursive computations:
// May cause stack overflow for large numbers
fun regularFibonacci(n: Int): Long {
return if (n <= 1) n.toLong()
else regularFibonacci(n - 1) + regularFibonacci(n - 2)
}
// Stack safe implementation
tailrec fun fibonacci(n: Int, a: Long = 0, b: Long = 1): Long {
return when (n) {
0 -> a
1 -> b
else -> fibonacci(n - 1, b, a + b)
}
}
2. Performance
Optimized to use constant stack space:
tailrec fun sum(n: Long, accumulator: Long = 0): Long {
return when (n) {
0L -> accumulator
else -> sum(n - 1, accumulator + n)
}
}
Common Use Cases
1. List Processing
sealed class List<out T> {
object Nil : List<Nothing>()
data class Cons<T>(val head: T, val tail: List<T>) : List<T>()
}
tailrec fun <T> length(list: List<T>, accumulator: Int = 0): Int {
return when (list) {
is List.Nil -> accumulator
is List.Cons -> length(list.tail, accumulator + 1)
}
}
2. Tree Traversal
data class TreeNode<T>(
val value: T,
val left: TreeNode<T>? = null,
val right: TreeNode<T>? = null
)
// Tail-recursive in-order traversal
tailrec fun <T> inOrderTraversal(
node: TreeNode<T>?,
stack: MutableList<TreeNode<T>> = mutableListOf(),
result: MutableList<T> = mutableListOf()
): List<T> {
return when {
node == null && stack.isEmpty() -> result
node == null -> {
val current = stack.removeAt(stack.lastIndex)
result.add(current.value)
inOrderTraversal(current.right, stack, result)
}
else -> {
stack.add(node)
inOrderTraversal(node.left, stack, result)
}
}
}
3. String Processing
tailrec fun reverseString(
str: String,
index: Int = str.length - 1,
accumulator: String = ""
): String {
return if (index < 0) accumulator
else reverseString(str, index - 1, accumulator + str[index])
}
Advanced Patterns
1. Mutual Recursion
class EvenOddChecker {
tailrec fun isEven(n: Int): Boolean {
return when (n) {
0 -> true
else -> isOdd(n - 1)
}
}
tailrec fun isOdd(n: Int): Boolean {
return when (n) {
0 -> false
else -> isEven(n - 1)
}
}
}
2. Continuation Passing Style
tailrec fun <T, R> traverse(
list: List<T>,
continuation: (List<T>, List<R>) -> List<R>,
accumulated: List<R> = emptyList()
): List<R> {
return when (list) {
is List.Nil -> continuation(list, accumulated)
is List.Cons -> traverse(
list.tail,
continuation,
accumulated + list.head
)
}
}
Best Practices
1. Accumulator Pattern
// Converting non-tail recursive to tail recursive using accumulator
tailrec fun gcd(a: Int, b: Int): Int {
return if (b == 0) a
else gcd(b, a % b)
}
tailrec fun power(base: Int, exponent: Int, accumulator: Int = 1): Int {
return when (exponent) {
0 -> accumulator
else -> power(base, exponent - 1, accumulator * base)
}
}
2. Stack Management
class StackSafeOperations {
tailrec fun processLargeList(
items: List<String>,
processed: MutableList<String> = mutableListOf()
): List<String> {
return when {
items.isEmpty() -> processed
else -> {
processed.add(items.first().uppercase())
processLargeList(items.drop(1), processed)
}
}
}
}
Common Pitfalls and Solutions
1. Non-Tail Recursive Patterns
// Not tail-recursive
fun badSum(list: List<Int>): Int {
return when (list) {
is List.Nil -> 0
is List.Cons -> list.head + badSum(list.tail) // Not tail-recursive
}
}
// Converted to tail-recursive
tailrec fun goodSum(list: List<Int>, acc: Int = 0): Int {
return when (list) {
is List.Nil -> acc
is List.Cons -> goodSum(list.tail, acc + list.head)
}
}
2. Multiple Recursive Calls
// Not tail-recursive due to multiple recursive calls
fun badFibonacci(n: Int): Long {
return if (n <= 1) n.toLong()
else badFibonacci(n - 1) + badFibonacci(n - 2)
}
// Converted to tail-recursive
tailrec fun goodFibonacci(
n: Int,
current: Long = 0,
next: Long = 1
): Long {
return when (n) {
0 -> current
else -> goodFibonacci(n - 1, next, current + next)
}
}
Conclusion
Tail recursion in Kotlin provides a powerful way to write recursive functions that are both stack-safe and efficient. Key points to remember:
- Use the
tailrec
modifier to ensure tail recursion optimization - Convert regular recursion to tail recursion using accumulators
- Ensure the recursive call is the last operation
- Consider tail recursion for processing large data structures
- Watch out for common pitfalls like multiple recursive calls
When used appropriately, tail recursion can help you write more efficient and safer recursive functions while maintaining the elegance of functional programming patterns.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.