Understanding the Bubble Sort Algorithm: A Step-by-Step Guide
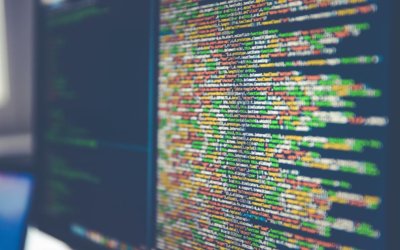
Categories:
9 minute read
Sorting algorithms are essential in computer science, as they are used to organize data in various applications like databases, search engines, and data analytics. Among the most fundamental and commonly taught sorting algorithms is Bubble Sort. While Bubble Sort is not the most efficient algorithm for large datasets, its simplicity makes it an excellent starting point for learning sorting algorithms.
In this blog post, we’ll explore what the Bubble Sort algorithm is, how it works, its advantages and disadvantages, and how it compares to other sorting algorithms. We’ll also discuss real-world applications and scenarios where Bubble Sort can still be useful despite its inefficiencies.
What is the Bubble Sort Algorithm?
Bubble Sort is a comparison-based sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. This process continues until the list is sorted. The algorithm gets its name because smaller elements “bubble” to the top (beginning) of the list, while larger elements “sink” to the bottom (end) with each pass through the list.
Key Characteristics of Bubble Sort:
In-place Sorting: Bubble Sort does not require any additional memory for sorting beyond the input array, making it an in-place sorting algorithm.
Stable Sorting: Bubble Sort is a stable sorting algorithm, meaning that it preserves the relative order of equal elements.
Simplicity: The algorithm is simple to understand and implement, making it a common choice for teaching the fundamentals of sorting.
How Does Bubble Sort Work?
Bubble Sort works by iterating through the list multiple times, comparing adjacent elements and swapping them if they are in the wrong order. This process continues until the list is fully sorted.
Step-by-Step Explanation of Bubble Sort
Let’s go through the Bubble Sort process with an example. Consider the following unsorted array:
[ [5, 3, 8, 4, 2] ]
First Pass:
Compare the first two elements (5 and 3). Since 5 > 3, we swap them: [ [3, 5, 8, 4, 2] ]
Compare the next two elements (5 and 8). No swap is needed because 5 < 8. [ [3, 5, 8, 4, 2] ]
Compare the next two elements (8 and 4). Since 8 > 4, we swap them: [ [3, 5, 4, 8, 2] ]
Compare the last two elements (8 and 2). Since 8 > 2, we swap them: [ [3, 5, 4, 2, 8] ] At the end of the first pass, the largest element (8) has “bubbled” to its correct position at the end of the list.
Second Pass:
Compare the first two elements (3 and 5). No swap is needed because 3 < 5. [ [3, 5, 4, 2, 8] ]
Compare the next two elements (5 and 4). Since 5 > 4, we swap them: [ [3, 4, 5, 2, 8] ]
Compare the next two elements (5 and 2). Since 5 > 2, we swap them: [ [3, 4, 2, 5, 8] ] At the end of the second pass, the second-largest element (5) has moved to its correct position.
Third Pass:
Compare the first two elements (3 and 4). No swap is needed because 3 < 4. [ [3, 4, 2, 5, 8] ]
Compare the next two elements (4 and 2). Since 4 > 2, we swap them: [ [3, 2, 4, 5, 8] ] At the end of the third pass, the third-largest element (4) is in its correct position.
Fourth Pass:
- Compare the first two elements (3 and 2). Since 3 > 2, we swap them: [ [2, 3, 4, 5, 8] ] Now the list is fully sorted, and no more passes are needed.
Pseudocode for Bubble Sort
Here’s the pseudocode for Bubble Sort to give a clearer idea of the algorithm:
BubbleSort(arr[], n)
for i = 0 to n-1 do
for j = 0 to n-i-2 do
if arr[j] > arr[j+1] then
Swap arr[j] and arr[j+1]```
In this pseudocode, the outer loop runs for each element in the array, while the inner loop compares and swaps adjacent elements.
## Time and Space Complexity of Bubble Sort
Bubble Sort is known for its simplicity, but it is not the most efficient sorting algorithm, especially for large datasets. Let’s break down its time and space complexity:
* **Time Complexity**:
* **Best Case**: The best-case time complexity occurs when the array is already sorted. In this case, Bubble Sort still iterates through the array once, but no swaps are made. The best-case time complexity is **O(n)**.
* **Worst Case**: In the worst case, where the array is sorted in reverse order, Bubble Sort must compare and swap each element in every pass. The worst-case time complexity is **O(n^2)**.
* **Average Case**: In the average case, Bubble Sort performs **O(n^2)** comparisons and swaps, making it inefficient for large datasets.
* **Space Complexity**: Bubble Sort is an in-place sorting algorithm, so it only uses **O(1)** additional memory, which is an advantage when memory is limited.
## Optimizing Bubble Sort
While Bubble Sort is generally not the most efficient algorithm, there are ways to optimize it:
Early Termination
In the worst case, Bubble Sort continues to make unnecessary passes even after the array is sorted. To avoid this, we can introduce a flag to detect if any swaps were made during a pass. If no swaps are made, the array is already sorted, and we can terminate the algorithm early.
Here’s the optimized pseudocode:
```bash
OptimizedBubbleSort(arr[], n)
for i = 0 to n-1 do
swapped = false
for j = 0 to n-i-2 do
if arr[j] > arr[j+1] then
Swap arr[j] and arr[j+1]
swapped = true
if swapped == false
break```
By adding the `swapped` flag, we ensure that the algorithm stops as soon as the array is sorted, potentially reducing the number of passes in the best-case scenario.
## Comparison with Other Sorting Algorithms
Bubble Sort vs. Selection Sort
**Selection Sort** is another simple sorting algorithm that repeatedly selects the smallest (or largest) element from the unsorted portion of the array and moves it to the sorted portion.
* **Time Complexity**: Both Bubble Sort and Selection Sort have a worst-case time complexity of **O(n^2)**, but Selection Sort often performs fewer swaps.
* **Stability**: Bubble Sort is stable, while Selection Sort is not. This means that Bubble Sort preserves the relative order of equal elements, whereas Selection Sort does not.
* **Practical Use**: Due to fewer swaps, Selection Sort may perform better than Bubble Sort in practice, but both algorithms are inefficient for large datasets.
Bubble Sort vs. Insertion Sort
**Insertion Sort** is a simple algorithm that builds the sorted array one element at a time by inserting each element into its correct position.
* **Time Complexity**: In the best case, when the array is nearly sorted, Insertion Sort has a time complexity of **O(n)**, making it more efficient than Bubble Sort, which always runs in **O(n^2)** in the worst case.
* **Stability**: Both Bubble Sort and Insertion Sort are stable.
* **Practical Use**: Insertion Sort is generally preferred over Bubble Sort, especially for small or nearly sorted arrays, because of its better performance in the average and best cases.
Bubble Sort vs. Quick Sort
**Quick Sort** is a highly efficient sorting algorithm that uses a divide-and-conquer approach to sort arrays.
* **Time Complexity**: Quick Sort has an average time complexity of **O(n log n)**, making it much faster than Bubble Sort for large datasets.
* **Space Complexity**: Quick Sort is also an in-place sorting algorithm, but its worst-case time complexity is **O(n^2)**. However, with proper pivot selection, this worst-case scenario can be avoided.
* **Practical Use**: Quick Sort is widely used in real-world applications due to its efficiency, while Bubble Sort is primarily used for educational purposes.
## Advantages of Bubble Sort
Despite its inefficiencies, Bubble Sort has a few advantages:
1. **Simplicity**
Bubble Sort is one of the simplest sorting algorithms to understand and implement. Its straightforward logic makes it an excellent choice for introducing sorting concepts to beginners.
2. **In-place Sorting**
Bubble Sort operates directly on the input array and does not require additional memory, making it an in-place sorting algorithm. This feature is beneficial in memory-constrained environments.
3. **Stability**
As a stable sorting algorithm, Bubble Sort maintains the relative order of equal elements, which can be important in certain applications, such as sorting records by multiple criteria.
# #
Disadvantages of Bubble Sort
Despite its simplicity, Bubble Sort has several drawbacks that limit its practical use:
1. **Inefficiency**
Bubble Sort has a time complexity of **O(n^2)**, making it highly inefficient for large datasets. The algorithm performs poorly compared to more advanced sorting techniques like Quick Sort, Merge Sort, and Heap Sort, especially when handling large arrays.
2. **Excessive Comparisons and Swaps**
Bubble Sort compares and swaps elements even if the array is already sorted or nearly sorted, leading to unnecessary operations. Although early termination can optimize the algorithm, it is still slower than other sorting methods for most cases.
## Real-World Applications of Bubble Sort
Given its inefficiency, Bubble Sort is rarely used in real-world applications where performance is a concern. However, there are a few scenarios where it may still be relevant:
* **Educational Purposes**: Bubble Sort is commonly taught in computer science courses as an introductory algorithm due to its simplicity.
* **Small Datasets**: For small arrays or lists where the size of the dataset is minimal, Bubble Sort can be a reasonable choice because its inefficiency is less noticeable for small inputs.
* **Nearly Sorted Data**: When the data is nearly sorted, Bubble Sort (with the early termination optimization) can sort the array efficiently, achieving **O(n)** time complexity.
## Conclusion
Bubble Sort is one of the most fundamental sorting algorithms and is often the first sorting technique introduced in computer science. While it is simple to understand and implement, Bubble Sort is not suitable for large datasets due to its **O(n^2)** time complexity. More advanced algorithms, such as Quick Sort or Merge Sort, are generally preferred for larger datasets or when performance is critical.
However, Bubble Sort’s stability and in-place sorting properties make it a useful teaching tool and an acceptable choice for small or nearly sorted datasets. By understanding Bubble Sort, you gain a foundation in sorting algorithms that will help you tackle more complex and efficient sorting techniques as you continue learning.