Val vs Var: Detailed Explanation in Kotlin Programming Language
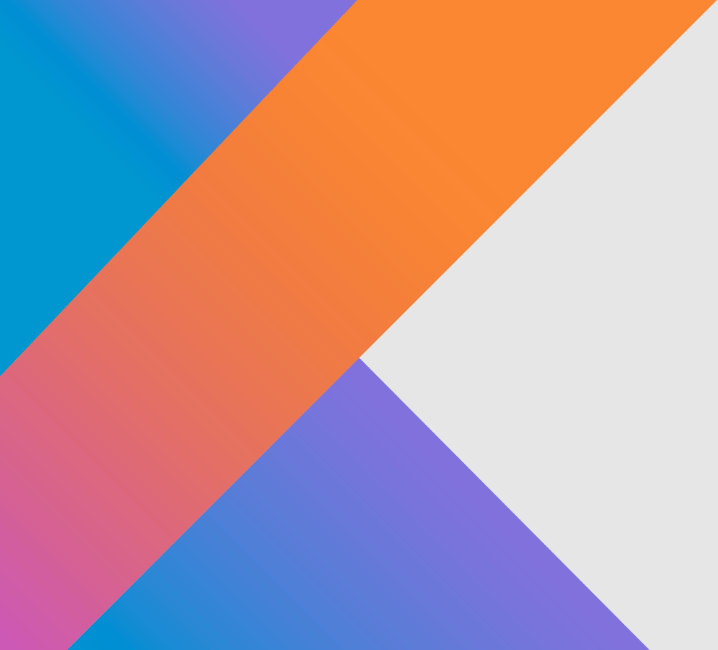
Categories:
3 minute read
Introduction
Kotlin, a statically typed programming language developed by JetBrains, has gained immense popularity due to its expressive and concise syntax. One of the fundamental concepts in Kotlin is variable declaration using val
and var
. Understanding the differences between these two keywords is essential for writing efficient and maintainable Kotlin code. In this article, we will explore the distinctions between val
and var
, their use cases, best practices, and real-world applications.
Understanding val
and var
Kotlin provides two primary ways to declare variables:
val
(Immutable variable) – Read-only variable whose value cannot be changed once assigned.var
(Mutable variable) – A variable whose value can be modified after initialization.
Both val
and var
require explicit or inferred type declaration, ensuring type safety in Kotlin programs.
val
(Immutable Variable)
val
stands for value, meaning it cannot be reassigned after its initial assignment. However, it is not equivalent to declaring a constant, as val
can hold objects with mutable properties.
Syntax
val name: String = "Kotlin"
In this example, name
is assigned "Kotlin"
, and any attempt to change it later will result in a compilation error.
Example
val age = 25
// age = 30 // This will cause a compilation error
var
(Mutable Variable)
var
stands for variable, meaning its value can be reassigned after declaration.
Syntax
var city: String = "New York"
city = "London" // Allowed
Here, the value of city
is initially "New York"
, but it can be reassigned to "London"
.
Example
var count = 10
count += 5 // Valid, count is now 15
Key Differences Between val
and var
Feature | val (Immutable) | var (Mutable) |
---|---|---|
Reassignable | No | Yes |
Performance | Generally better | Slightly less efficient |
Safety | Safer, prevents unintended modifications | May introduce unexpected changes |
Use Case | Constants, function results, and thread-safe programming | Variables that change frequently |
When to Use val
vs var
When to Use val
- Immutable Data Handling: When you want to ensure a variable’s value remains constant.
- Thread Safety:
val
helps avoid race conditions in multithreading. - Better Readability and Maintainability: Code is easier to understand when values do not change unexpectedly.
- Performance Optimization: Optimizations are possible as the compiler knows the value won’t change.
When to Use var
- Changing Values Over Time: When the variable represents a dynamic value.
- Loop Counters and Accumulators:
var
is useful for loop iterations and counters. - Mutable Data Structures: When working with collections where items need to be modified.
Example Use Cases
Using val
for Constants
val PI = 3.14159
val appName = "KotlinApp"
These values will never change, making val
the best choice.
Using var
for Dynamic Data
var score = 0
score += 10 // Incrementing score dynamically
Since score
needs to change, var
is appropriate.
val
with Mutable Objects
Although val
prevents reassignment, it does not make objects immutable.
val person = mutableListOf("Alice", "Bob")
person.add("Charlie") // Allowed, but person itself cannot be reassigned
Here, person
remains the same reference, but its contents can be modified.
Best Practices
- Prefer
val
over ********var
: Useval
unless mutation is necessary. - Use meaningful names: Variables should clearly indicate their purpose.
- Avoid unnecessary mutability: Too many
var
declarations can make debugging difficult.
Conclusion
Understanding the differences between val
and var
is fundamental in Kotlin programming. val
is ideal for ensuring immutability, enhancing performance, and reducing bugs, while var
is useful when values need to change dynamically. By following best practices and choosing the right variable type, developers can write clean, efficient, and maintainable Kotlin code.
Additional Resources
- Kotlin Documentation
- Effective Kotlin - A collection of best practices and guidelines for Kotlin development.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.