Variables and Data Types in Kotlin
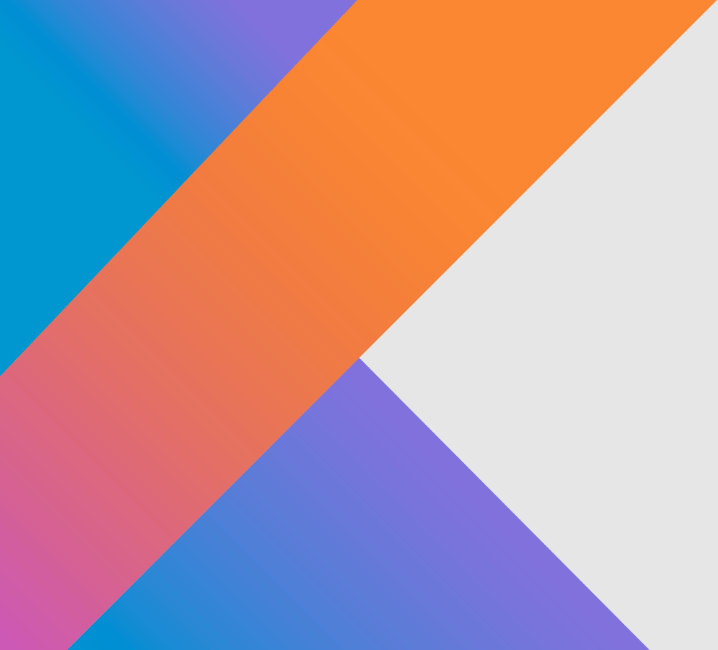
Categories:
2 minute read
Introduction
Kotlin is a statically typed programming language developed by JetBrains. It is widely used for Android development, backend applications, and even frontend development. Understanding Kotlin’s basic syntax, particularly variables and data types, is essential for writing efficient and robust applications. This article provides a comprehensive guide on Kotlin variables, types, and best practices.
Variables in Kotlin
Kotlin supports two types of variables:
- Immutable Variables (
val
): These variables are read-only and cannot be reassigned once initialized. - Mutable Variables (
var
): These variables can be reassigned.
Declaring Variables
Immutable (val
)
val name: String = "Kotlin"
val age: Int = 25
Once assigned, the values of val
variables cannot be changed.
Mutable (var
)
var city: String = "New York"
city = "London" // This is allowed
Unlike val
, var
allows reassignment.
Type Inference
Kotlin supports type inference, meaning you do not always need to explicitly specify the type.
val language = "Kotlin" // Compiler infers type as String
var count = 10 // Compiler infers type as Int
Data Types in Kotlin
Kotlin provides various built-in data types categorized into the following:
1. Numeric Data Types
Kotlin supports different types of numbers:
- Integers:
Byte
,Short
,Int
,Long
- Floating Point Numbers:
Float
,Double
Examples
val byteValue: Byte = 8
val shortValue: Short = 1000
val intValue: Int = 100000
val longValue: Long = 1000000000L
val floatValue: Float = 98.6F
val doubleValue: Double = 123.456
2. Boolean Type
The Boolean
type represents two values: true
or false
.
val isKotlinFun: Boolean = true
3. Character and String Types
Char
: Represents a single character.String
: Represents a sequence of characters.
Examples
val letter: Char = 'K'
val message: String = "Hello, Kotlin!"
String Templates
Kotlin supports string interpolation using the $
symbol.
val name = "Kotlin"
println("Hello, $name")
4. Arrays
An array is a collection of elements of the same type.
val numbers = arrayOf(1, 2, 3, 4, 5)
println(numbers[0]) // Outputs: 1
5. Collections
Kotlin provides built-in support for collections such as Lists, Sets, and Maps.
val list = listOf("Apple", "Banana", "Cherry")
val mutableList = mutableListOf("Dog", "Cat")
Type Conversion
Explicit type conversion is required in Kotlin as it does not perform implicit type conversions.
val intValue: Int = 10
val doubleValue: Double = intValue.toDouble()
println(doubleValue) // Outputs: 10.0
Conclusion
Understanding Kotlin’s variable declaration, data types, and type inference is crucial for writing clean and effective code. By leveraging Kotlin’s type system, developers can build safe and expressive applications. In the next steps, you can explore advanced Kotlin concepts like control flow and functions.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.