While Loops in Kotlin
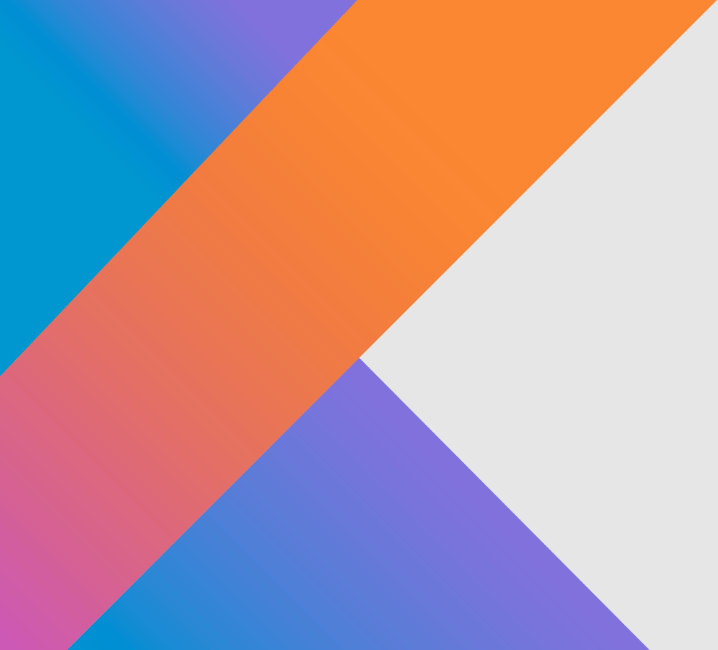
Categories:
5 minute read
While loops are fundamental control flow structures in Kotlin that allow you to execute a block of code repeatedly as long as a specific condition remains true. In this comprehensive guide, we’ll explore the various aspects of while loops in Kotlin, including their syntax, use cases, and best practices.
Basic While Loop Syntax
In Kotlin, there are two types of while loops: the standard while
loop and the do-while
loop. Let’s examine both in detail.
Standard While Loop
The basic syntax of a while loop is:
while (condition) {
// Code block to be executed
}
Here’s a simple example:
var counter = 1
while (counter <= 5) {
println("Counter: $counter")
counter++
}
Do-While Loop
The do-while loop executes the code block at least once before checking the condition:
do {
// Code block to be executed
} while (condition)
Example:
var number = 1
do {
println("Number: $number")
number++
} while (number <= 5)
Key Differences Between While and Do-While
Understanding when to use each type of while loop is crucial for writing effective code. Here are the main differences:
Condition Checking:
- While loop: Checks condition before executing the code block
- Do-while loop: Checks condition after executing the code block
Minimum Execution:
- While loop: May never execute if the initial condition is false
- Do-while loop: Always executes at least once
Example demonstrating the difference:
// While loop with false condition
var x = 10
while (x < 10) {
println("This will never be printed")
}
// Do-while loop with false condition
var y = 10
do {
println("This will be printed once")
} while (y < 10)
Common Use Cases for While Loops
Let’s explore some practical applications of while loops in Kotlin.
1. Input Validation
While loops are excellent for input validation scenarios:
fun readValidAge(): Int {
var age: Int
do {
println("Enter your age (1-120):")
age = readLine()?.toIntOrNull() ?: 0
} while (age !in 1..120)
return age
}
2. Processing Data Streams
While loops are useful for processing data until a certain condition is met:
fun processDataStream(stream: DataInputStream) {
while (stream.available() > 0) {
val data = stream.readByte()
// Process the data
}
}
3. Game Loops
While loops are commonly used in game development:
fun gameLoop() {
var isGameRunning = true
while (isGameRunning) {
updateGameState()
renderGraphics()
handleInput()
if (isGameOver()) {
isGameRunning = false
}
}
}
Advanced Techniques and Best Practices
1. Using Labels with While Loops
Kotlin supports labeled breaks and continues in while loops:
outerLoop@ while (true) {
var counter = 0
while (counter < 5) {
if (someCondition()) {
break@outerLoop // Breaks out of the outer loop
}
counter++
}
}
2. Infinite Loops with Control
Sometimes you need an intentional infinite loop with controlled exit conditions:
fun processQueue(queue: Queue<Task>) {
while (true) {
val task = queue.poll() ?: break
processTask(task)
}
}
3. Using Sequences with While Loops
Kotlin’s sequences can be effectively combined with while loops:
fun generateFibonacci(): Sequence<Int> = sequence {
var terms = Pair(0, 1)
while (true) {
yield(terms.first)
terms = Pair(terms.second, terms.first + terms.second)
}
}
Performance Considerations and Optimization
When working with while loops, consider these performance aspects:
1. Condition Evaluation
Ensure that the condition check is as efficient as possible:
// Less efficient
while (calculateComplexCondition()) {
// Loop body
}
// More efficient
val condition = calculateComplexCondition()
while (condition) {
// Loop body
}
2. Resource Management
Properly manage resources within while loops:
var reader: BufferedReader? = null
try {
reader = BufferedReader(FileReader("file.txt"))
var line: String?
while (reader.readLine().also { line = it } != null) {
// Process line
}
} finally {
reader?.close()
}
Common Pitfalls and How to Avoid Them
1. Infinite Loops
Ensure your while loops have a clear exit condition:
// Potential infinite loop
var counter = 0
while (counter < 10) {
println(counter)
// Forgot to increment counter
}
// Correct implementation
var counter = 0
while (counter < 10) {
println(counter)
counter++
}
2. Off-by-One Errors
Be careful with boundary conditions:
// Incorrect implementation
var i = 1
while (i <= 5) {
println(i)
i += 2
} // Prints: 1, 3, 5
// Correct implementation for even numbers
var i = 0
while (i <= 4) {
println(i)
i += 2
} // Prints: 0, 2, 4
3. Unnecessary While Loops
Sometimes a for loop or other construct might be more appropriate:
// Less idiomatic
var index = 0
while (index < list.size) {
println(list[index])
index++
}
// More idiomatic
for (item in list) {
println(item)
}
Best Practices for While Loop Usage
Clear Exit Conditions: Always ensure your while loops have clear and achievable exit conditions.
Appropriate Loop Choice: Choose the right type of loop for your use case:
- Use
while
when you don’t know how many iterations you need - Use
do-while
when you need at least one iteration - Consider using
for
loops for known collections or ranges
- Use
Loop Variables: Keep loop control variables simple and clearly named:
var attemptCount = 0
while (attemptCount < maxAttempts) {
if (tryOperation()) {
break
}
attemptCount++
}
Conclusion
While loops in Kotlin are powerful control flow structures that, when used correctly, can help you write clean and efficient code. Understanding the differences between while and do-while loops, knowing when to use each, and being aware of common pitfalls will help you write better Kotlin programs. Remember to always consider the readability and maintainability of your code when choosing between different loop constructs, and be mindful of performance implications in critical sections of your application.
By following the best practices and patterns outlined in this guide, you’ll be better equipped to use while loops effectively in your Kotlin projects, whether you’re building simple scripts or complex applications.
Feedback
Was this page helpful?
Glad to hear it! Please tell us how we can improve.
Sorry to hear that. Please tell us how we can improve.